In this topic, we will discuss about prior knowledge of JavaScript for Lightning Web Component
- Var, Let & const keywords
- Spread Operator
- De-structuring
- String Interpolation
- Array Functions
- Use of Promise
- Arrow Function
- Event
Var, Let & const keywords
var keyword
1. It can be updated and re-declare
var myName = "Sachin"
console.log(myName) // output :- Sachin
var myName = "Sachin Jha"
console.log(myName) // output :- Sachin Jha
2. Automatically bind with window property if its not inside any block or function
var bind = 'Hello, hi !!!'
console.log(window.bind) // output :- Hello, hi !!!
3. It support on function scope or global scope
function myFunScope(){
var varFunScope = 'Var Function Scope'
console.log(varFunScope)
}
myFunScope()
console.log(varFunScope) //---> (will give you an error)
4. Does no Support Block level scope
if(true){
var blkScope = 'Var Block Scope'
console.log(blkScope)
}
console.log(blkScope)
/* output:- Var Block Scope
Var Block Scope */
let keyword
let myName = "Sachin"
console.log(myName)
let myName = "Sachin Jha"
console.log(myName) // SyntaxError: 'myName' has already been declared
2. let support function and block level scope
function myLetFunScope(){
let letFunScope = 'let Function Scope'
console.log(letFunScope)
}
myLetFunScope()
//console.log(letFunScope) //---> (will give you an error)
3. It support global level scope but does not create property on global object
let myScope = 'window scope'
console.log(window.myScope) // will give you undefined
const keyword
const name = 'Sachin'
name = 'Sachin Jha'
console.log(name) // will give you an error
2. Support function and block level scope
function myLetFunScope(){
const letFunScope = 'const Function Scope'
console.log(letFunScope)
}
myLetFunScope()
//console.log(letFunScope) //---> (will give you an error)
3. It support global level scope but does not create property on global object
const a = 'a'
console.log(this.a) // undefined
Spread (…) Operator
The Spread operator allows an iterable to expand in places where 0+ arguments are expected. It is mostly used in the variable array where there is more than 1 value is expected. It allows us the privilege to obtain a list of parameters from an array.
- Expanding String : convert list into array
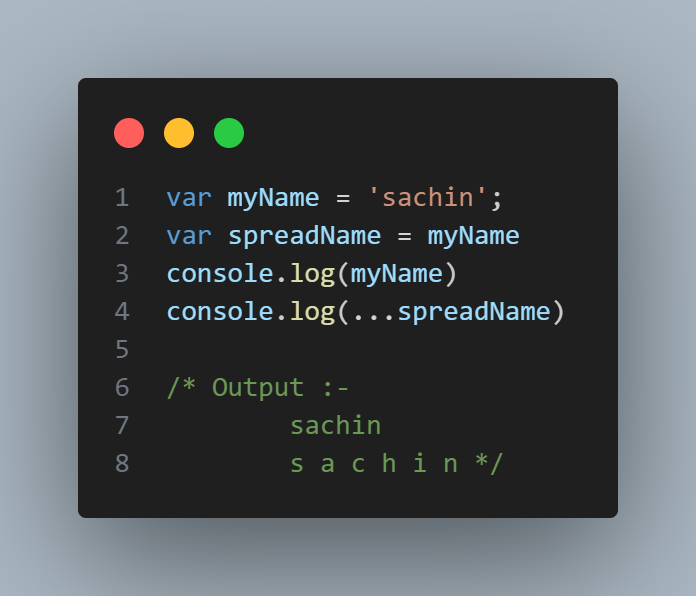
2. Combining Arrays : add value to array
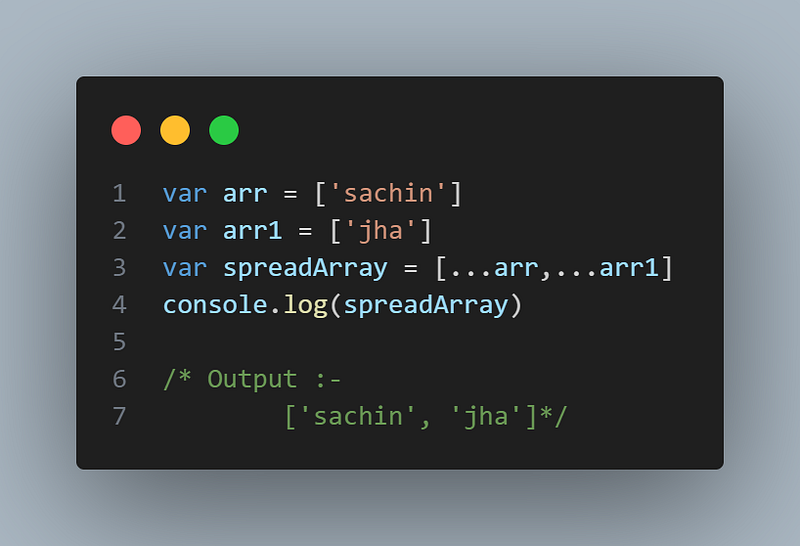
3. Combining Object : add value to Object
If left and right side spread operator has same property the right one will override it
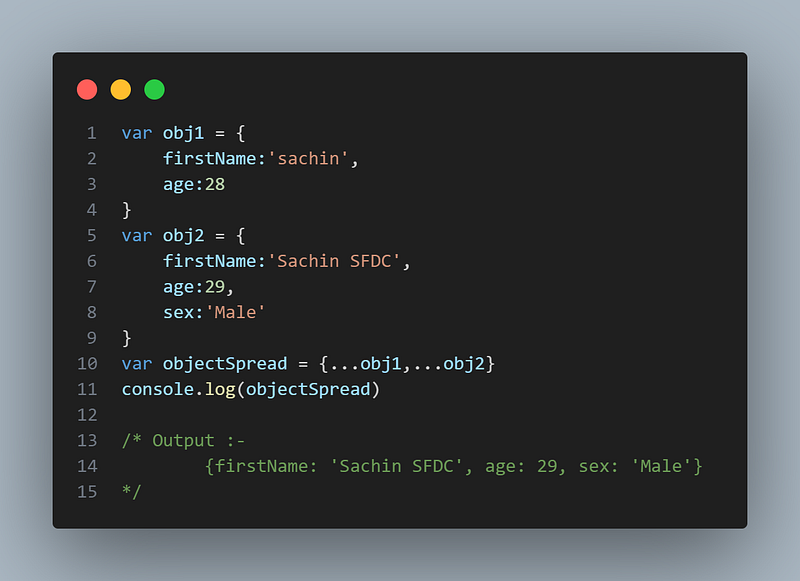
4. Creating new shallow copy of Array and object
Using spread operator, the previous value of array will not be affected if you push
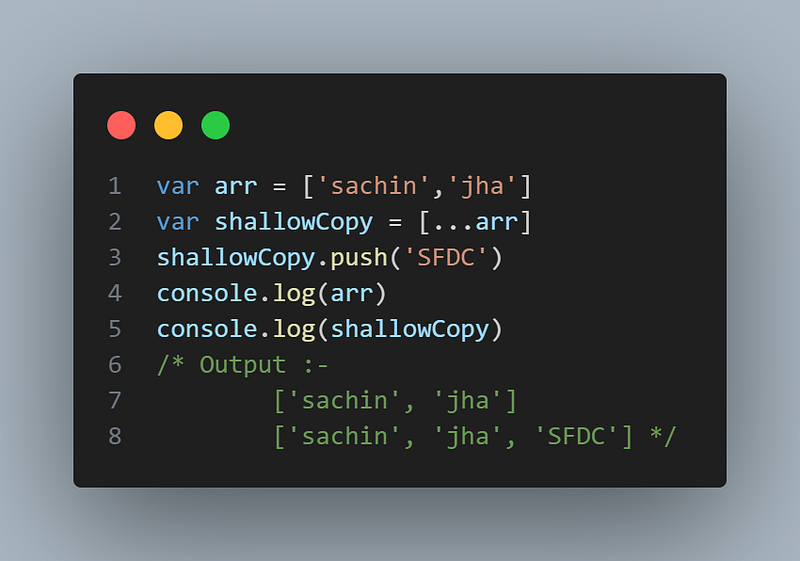
De-structuring
Array Destructuring
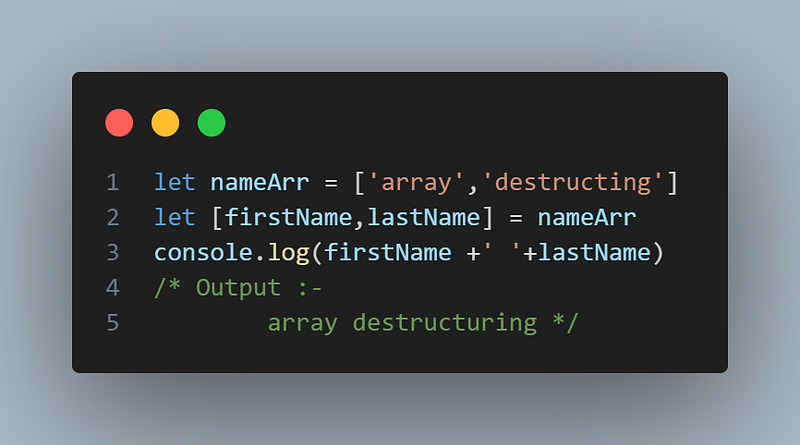
Object Destructuring
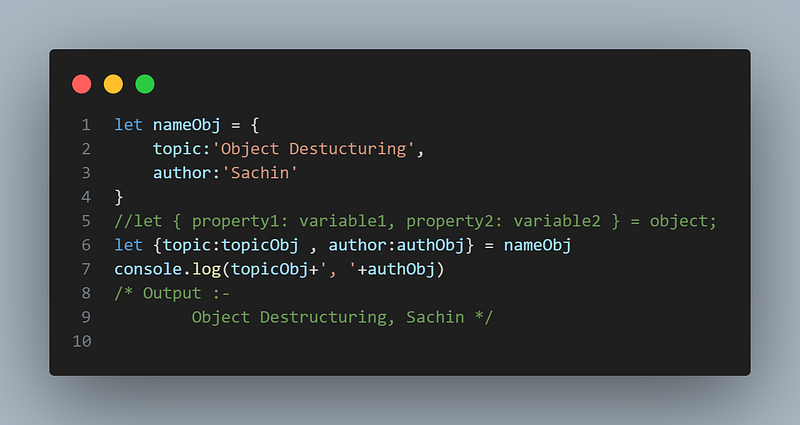
another way to assign
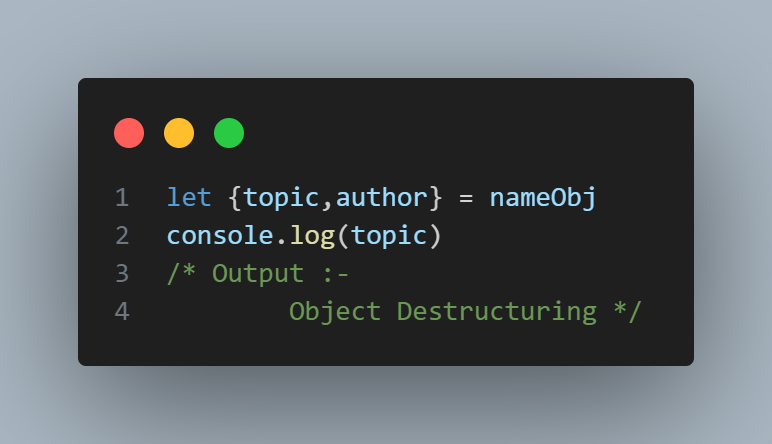
String Interpolation
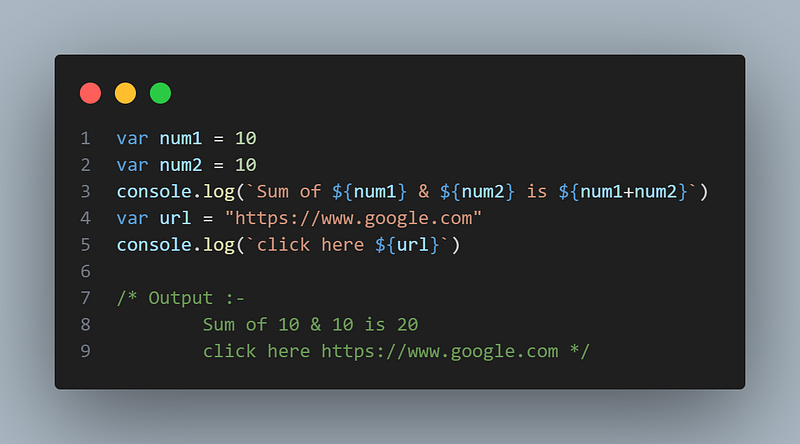
Array Function
syntax :-
arr.methodName(function(currentItem, index, array){
//return something
})
- map() method creates a new array with the results of calling a function for every array element.
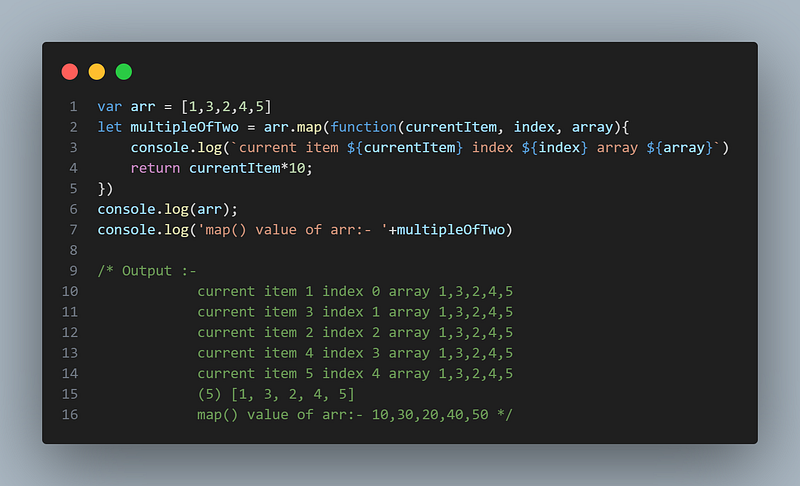
2. every() return true or false if every statement satisfied condition
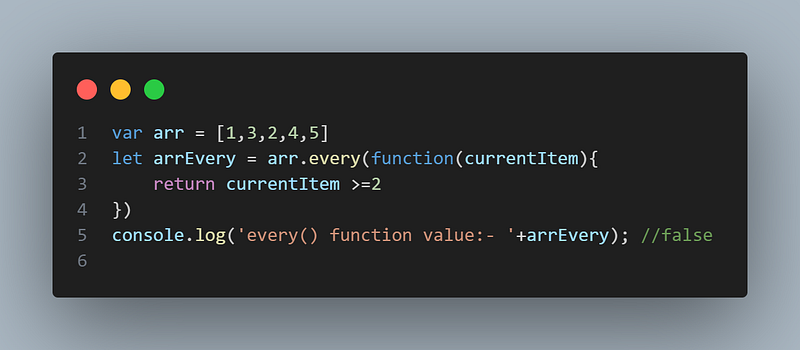
3. some() return true if at least one array item satisfied cond ition
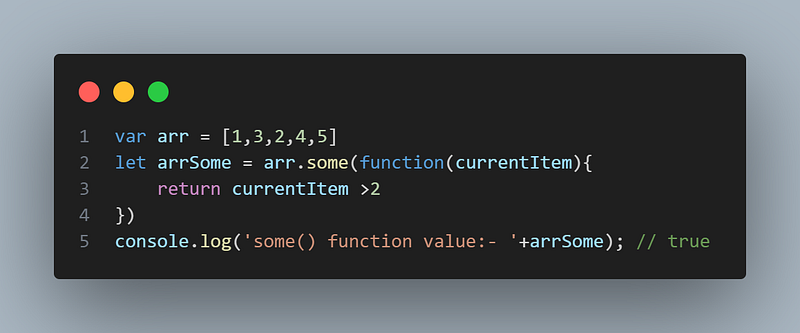
4. filter() return new array with filtered value
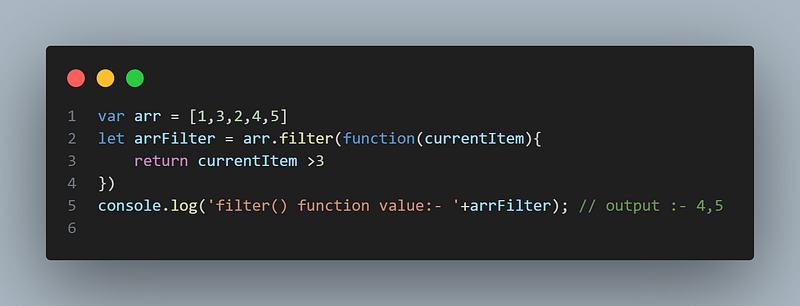
5. reduce() this function reduce array to a single value
syntax:-
arr.reduce(function(total, currentItem, index, array){
return something
}, initialValue)
whatever is your initial value is that would be in total value
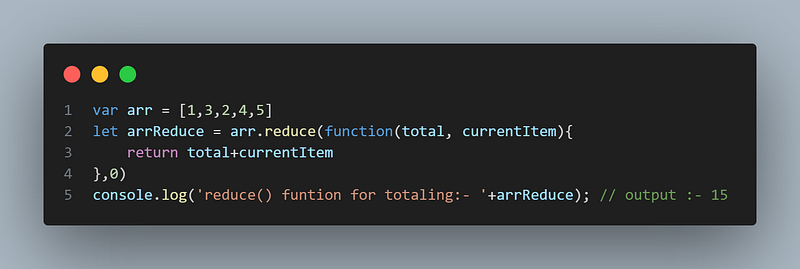
6. sort() used to sort elements in desc or asc
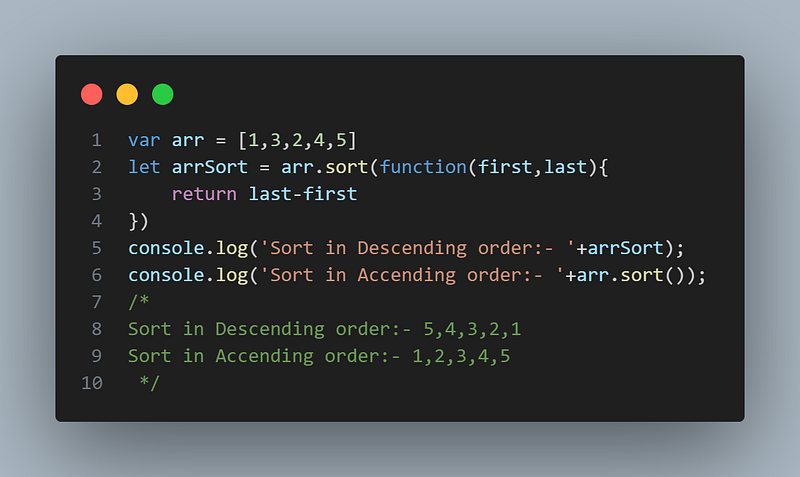
Use of Promise
Promise is an object and mostly used to handle async process.
To handle promise, we use .then() method to extract data and .catch() to handle error (if any).
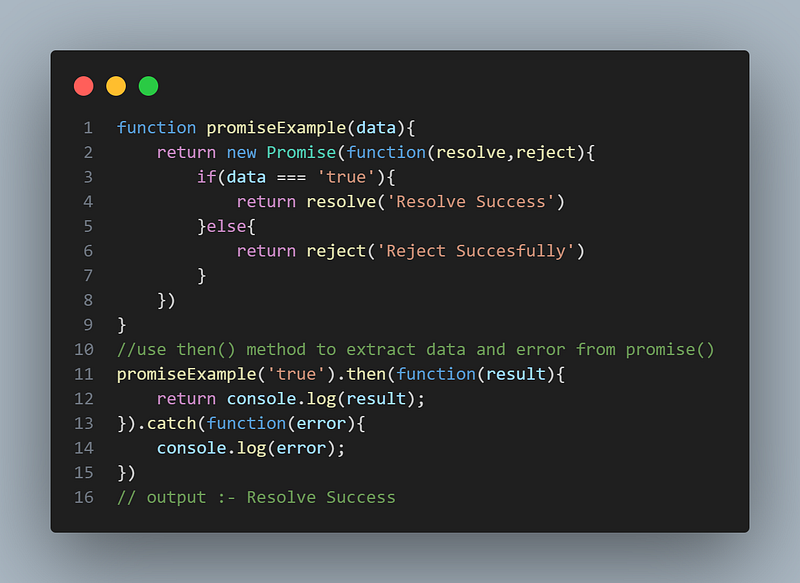
Fetching data from server
fetch() method is used to call service
Server call is always a promise call that’s why we use then() to get data
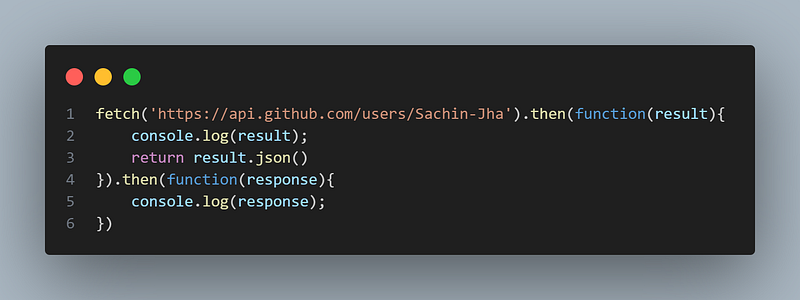
Arrow Function
Arrow Function is concise way of writing Javascript functions in shorter way.
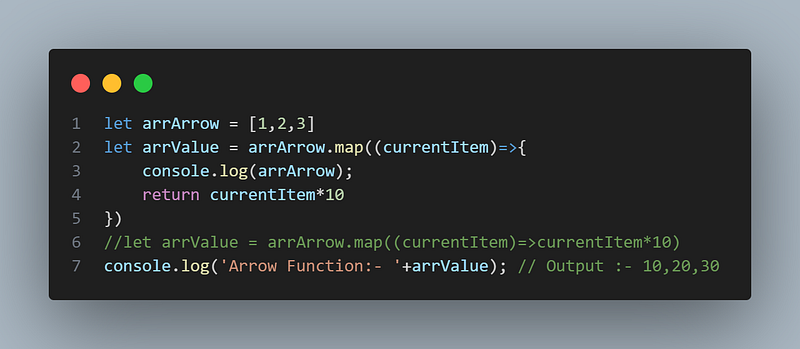
Event
Event Handler :-
when we provide event in HTML, event always begin with “on” eg onClick, onChange etc. It has predifined event available in HTML
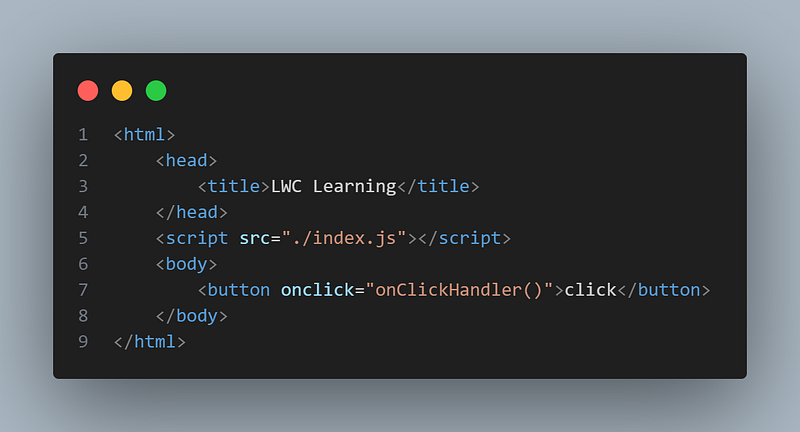
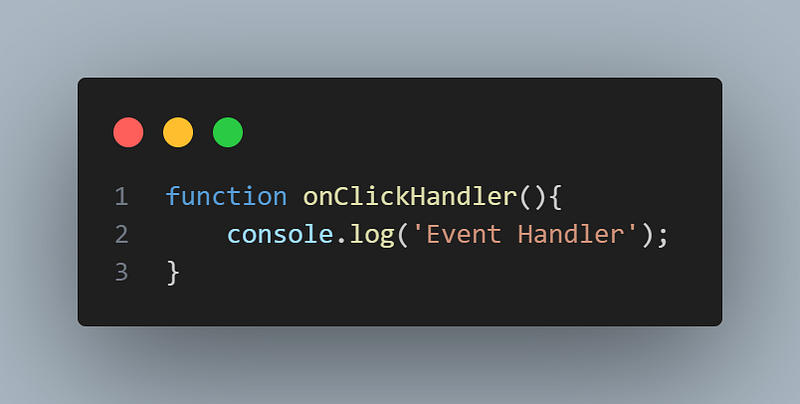
Event Listener :-
In Event Listener, we have two methods
1) addEventListener(‘eventName’, handler)
2) removeEventListener(‘eventName’, handler)
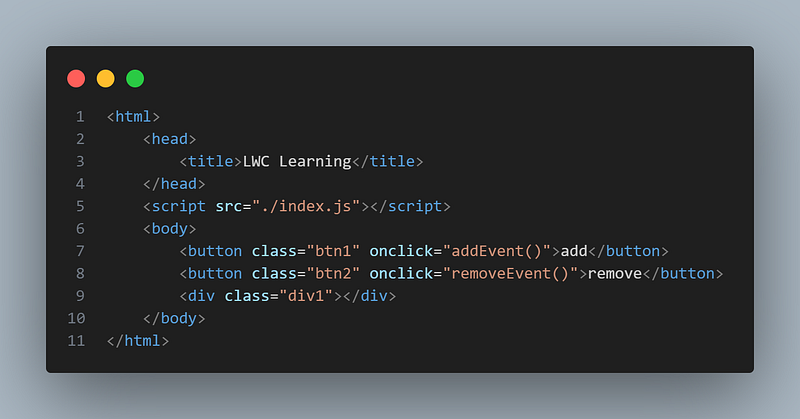
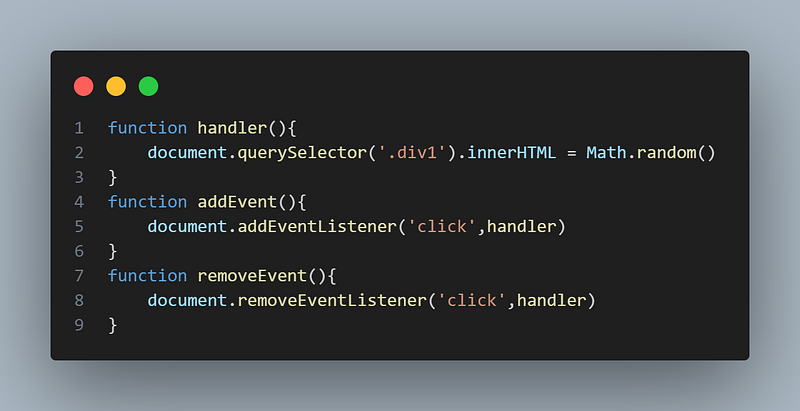
Custom Event :-
You can create custom event with the help of CustomeEvent constructor
After creating Custom Event, we need to dispatch event with help of dispatchEvent() method.
Once dispatching event done, we have to handle a listener, so we have to add addEventListener() method.
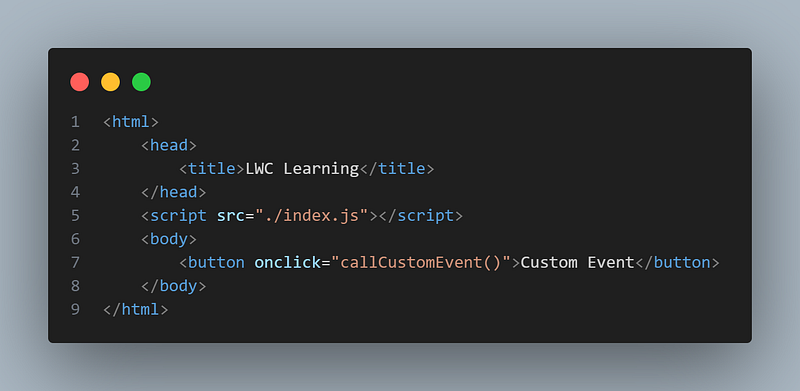
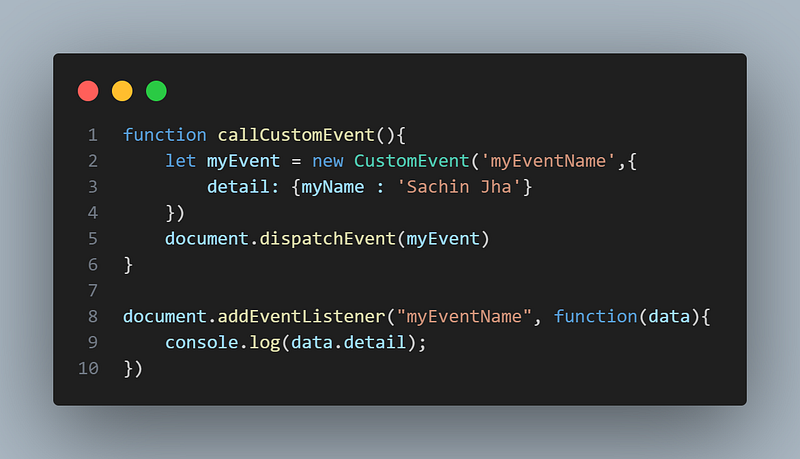
Follow me on linkedIn and Twitter for such more related post
Comments
Post a Comment