In this topic we will cover how LWC component communicate with each other
There are four ways to communicate a component
- Parent to Child communication
- Child to Parent communication
- Independent Component communication using Pub-Sub Model (Old technique)
- Cross Framework (Across VF page, Aura and LWC using lightning Messaging Service)
Parent to Child communication
To make parent to child communication, here are below steps
- Make a component composition, In parent class I have access child component like <c-component-communication-child>
- In child component, we have to create a public property which will hold parent data.
- To make public property, use @api decorator to create public property.
- To define child component public property in parent component, we write as with hyphen(-) sign. eg. if child component has @api userDetails then in parent component we have to write like user-details.
There are 4 ways to communicate Parent to Child
A. Passing Primitive data to child
B. Passing Non-Primitive data to child
C. Passing data to child on action event
D. Calling child method to parent
Passing Primitive data to child
— — — — — — — — — — — — — — — —
Parent component
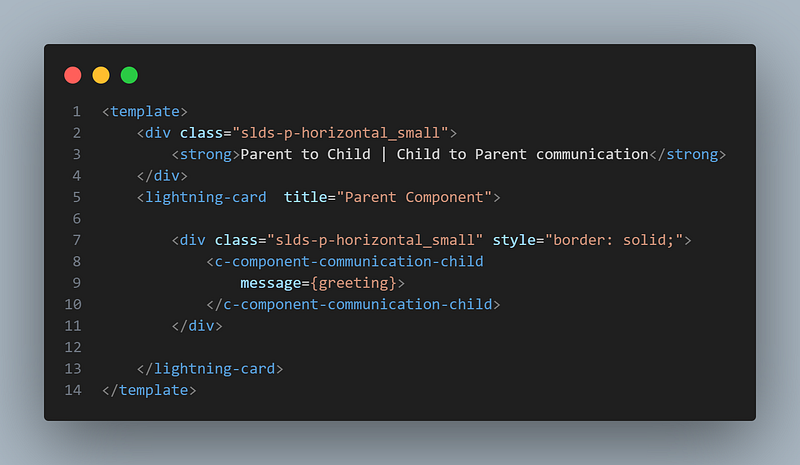
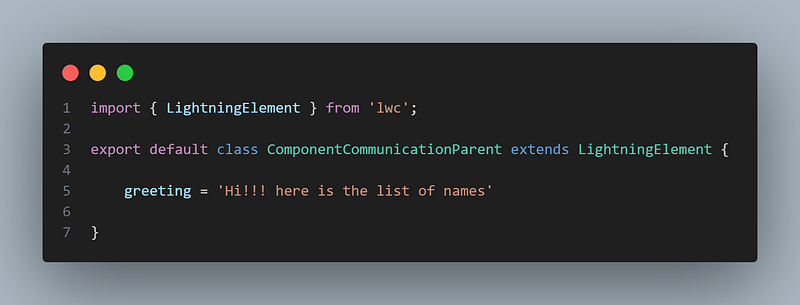
Child Component
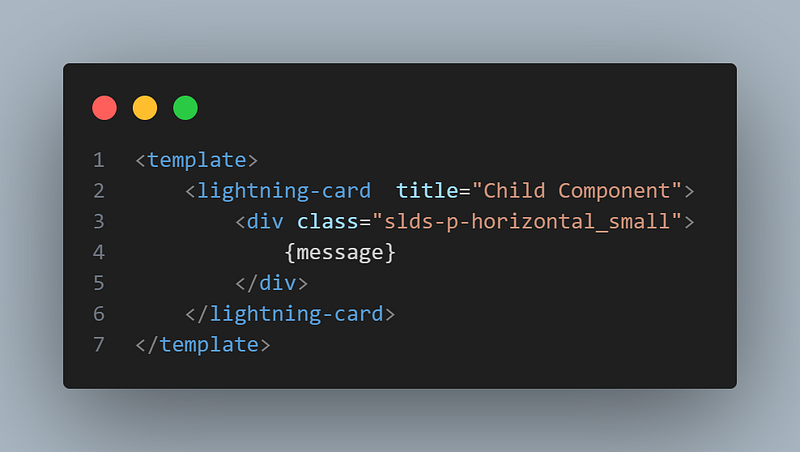
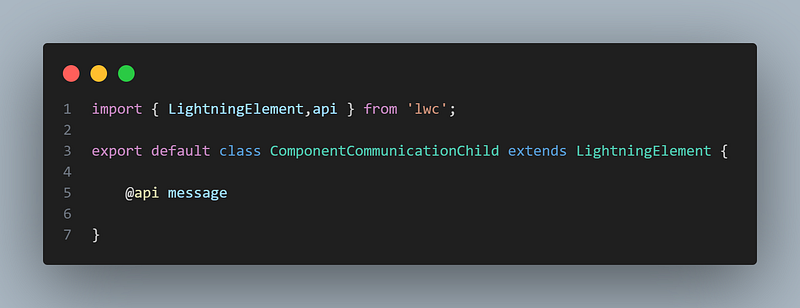
Result
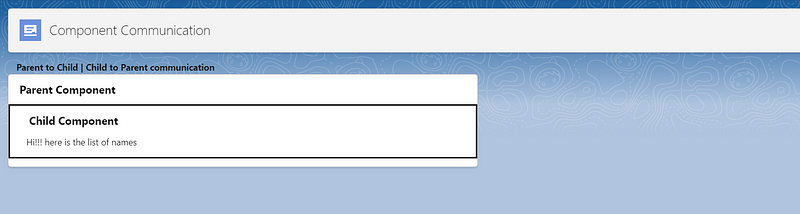
Passing Non-Primitive data to child
— — — — — — — — — — — — — — — — — — — —
Parent component
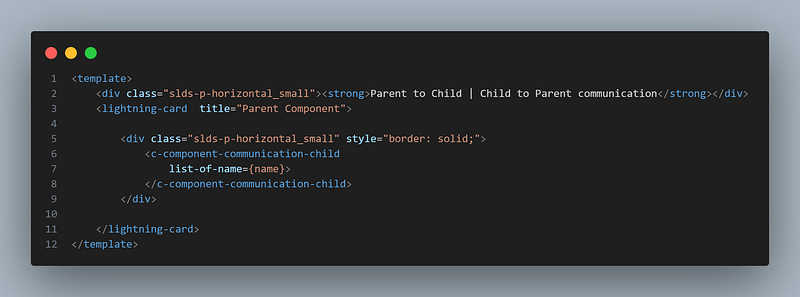
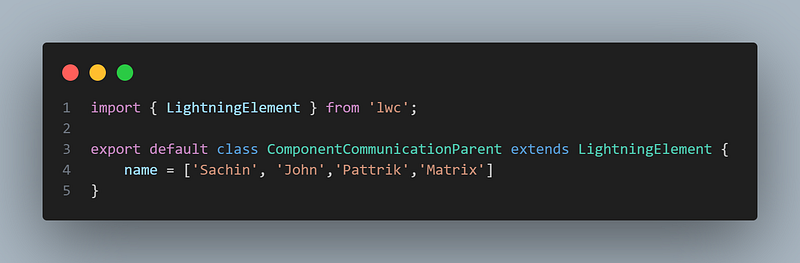
Child Component
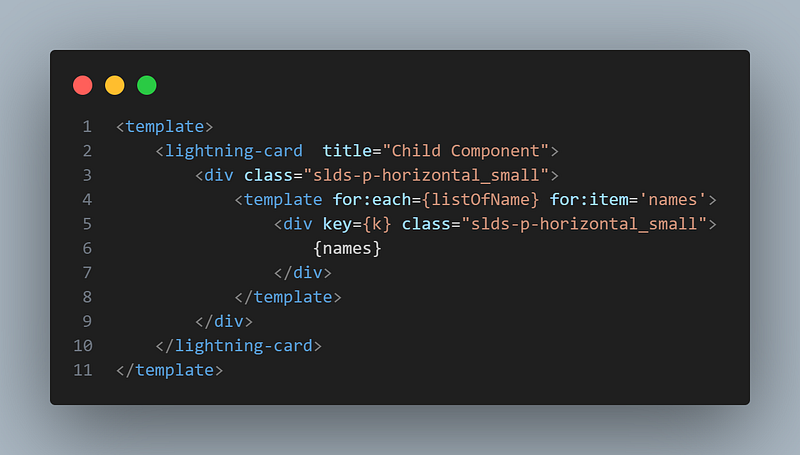
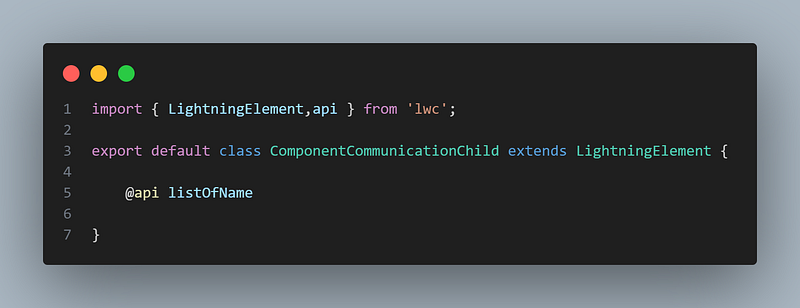
Result
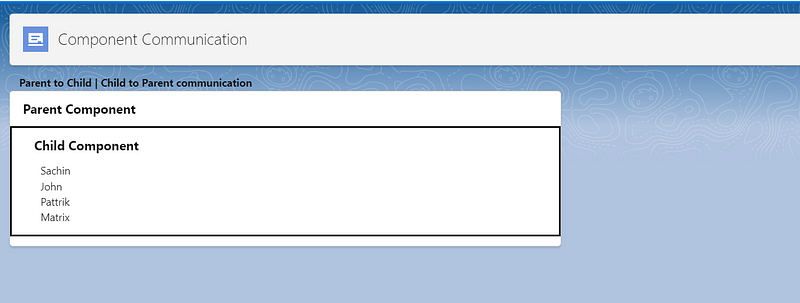
Passing data to child on action event
— — — — — — — — — — — — — — — — — — — — —
Parent component
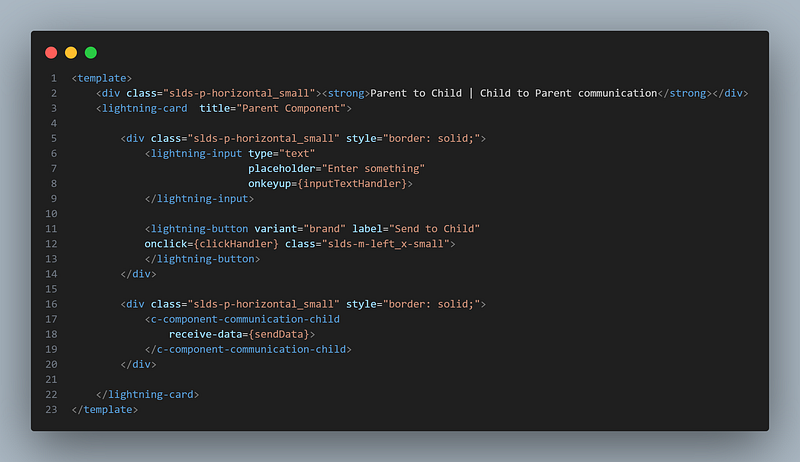
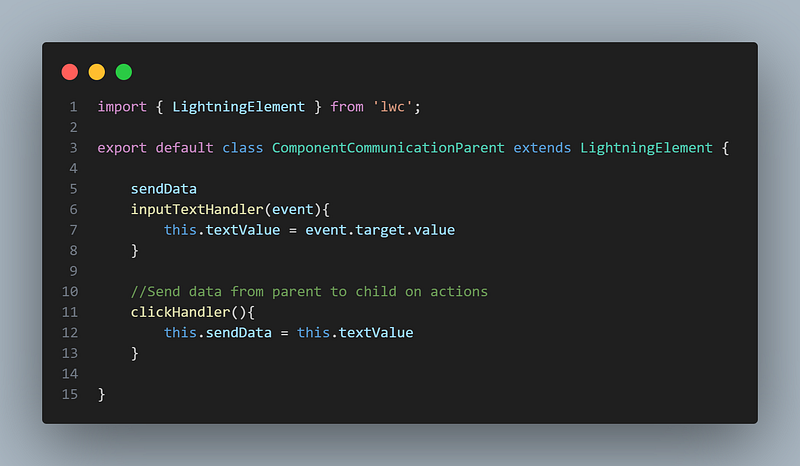
Child Component
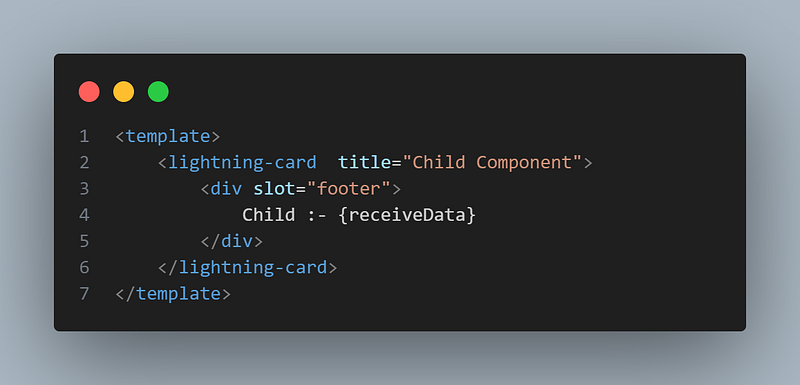
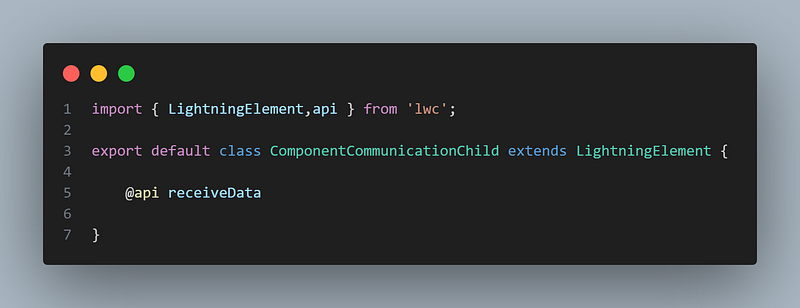
Result
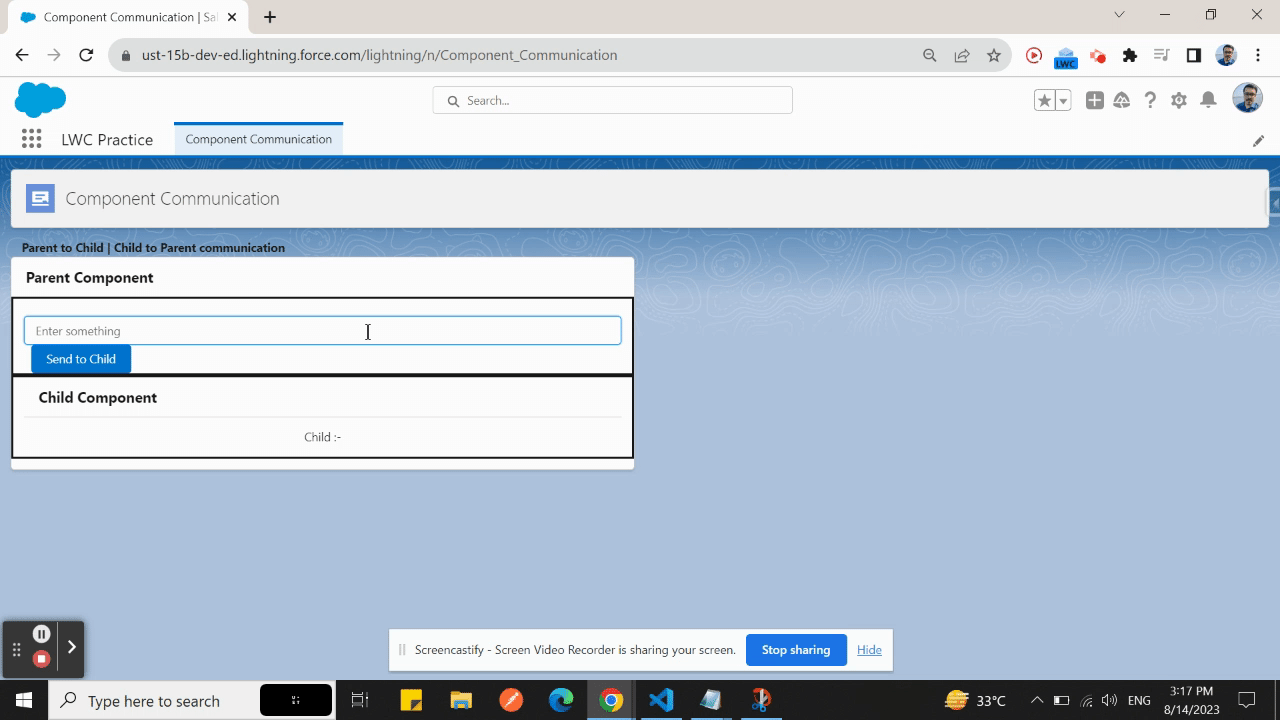
Calling child method to parent
— — — — — — — — — — — — — — — — — —
By using this.template.querySelector(), you can call child component method by passing child component name in querySelector parameter
eg:- this.template.querySelector(‘c-component-communication-child’).childMethod().
Parent Component
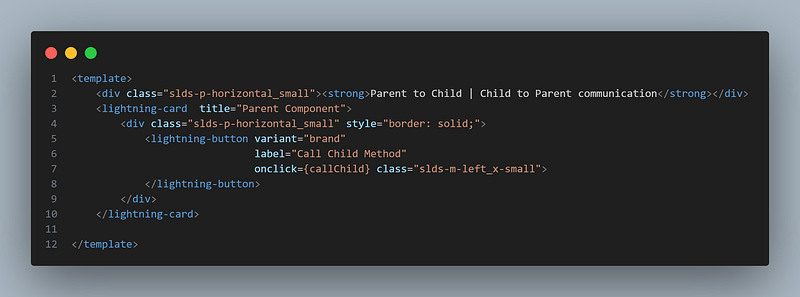
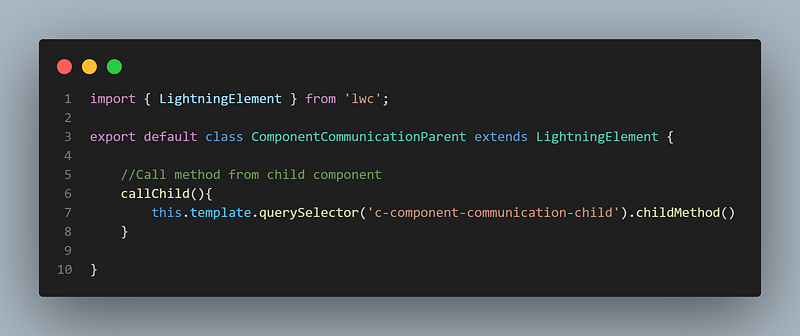
Child Component
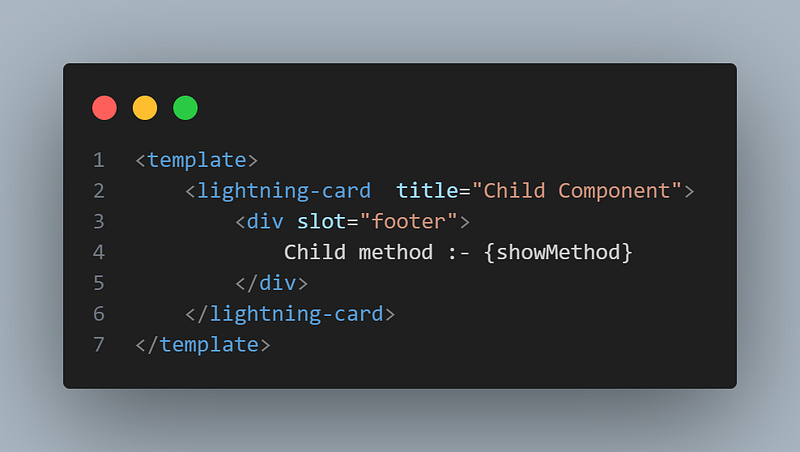
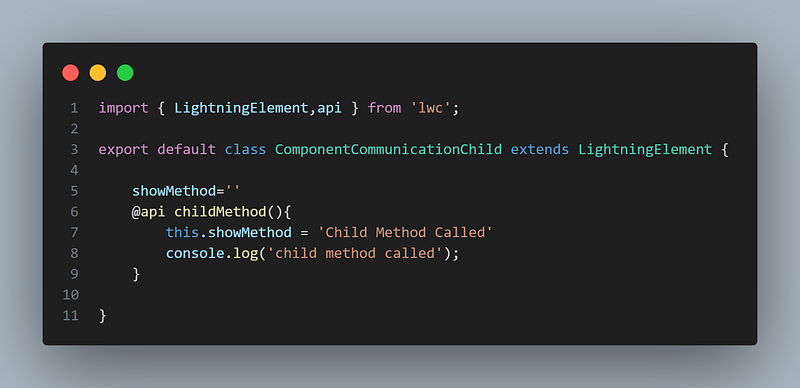
Result
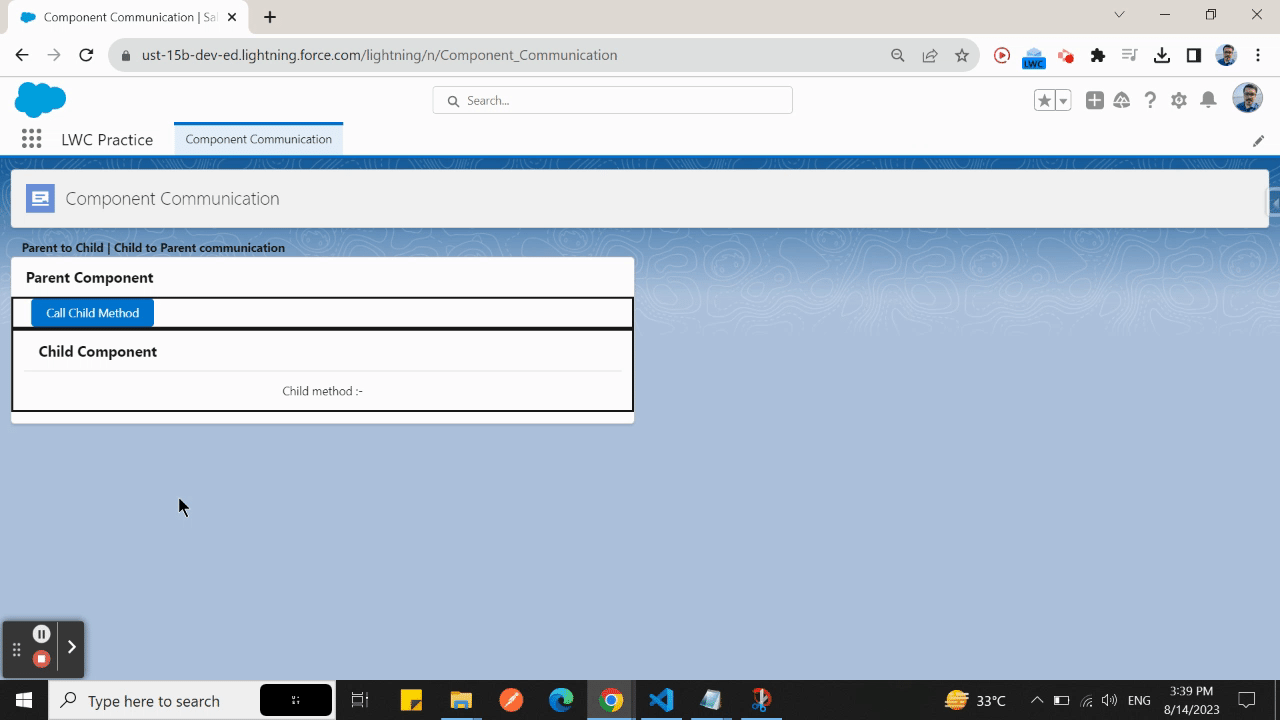
Child to Parent communication
To make child to parent communication, we have to use Custom Events and dispatch that event, but before we move to Custom Events, lets understand first what is Events.
What is Events?
Any interaction with JS and HTML are handled through Event. When the user or the browser wants to play with page when the page loads, it is called an event. All events are prefix with ‘on’ keyword.
For eg. onclick, onchange, onkeyup etc.
Create and Dispatch Custom Event
To create custom event, use CustomEvent() constructor. It has one required parameter, which is a string indicating the event type.
To fire Custom Event, use EventTarget.dispatchEvent().
In LWC, EventTarget is this.
Parent Component
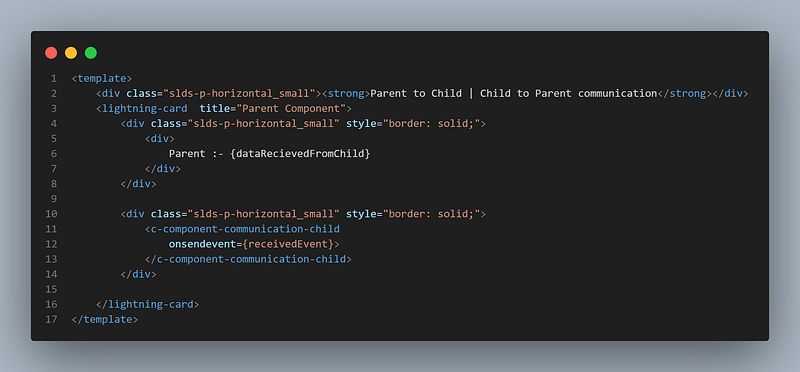
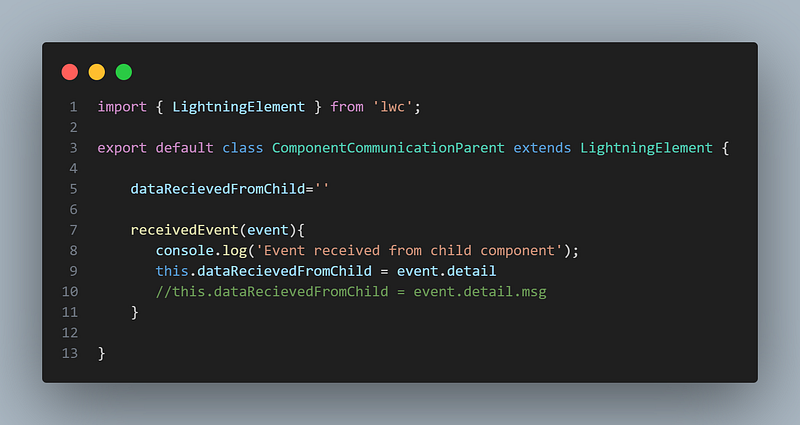
Child Component
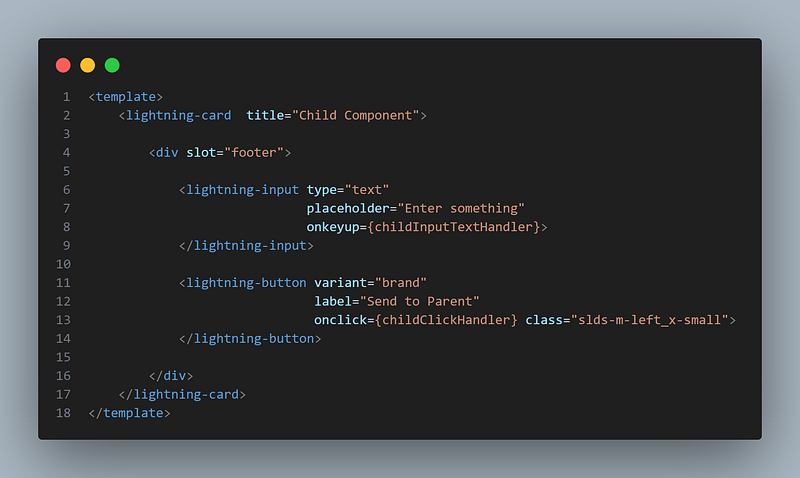
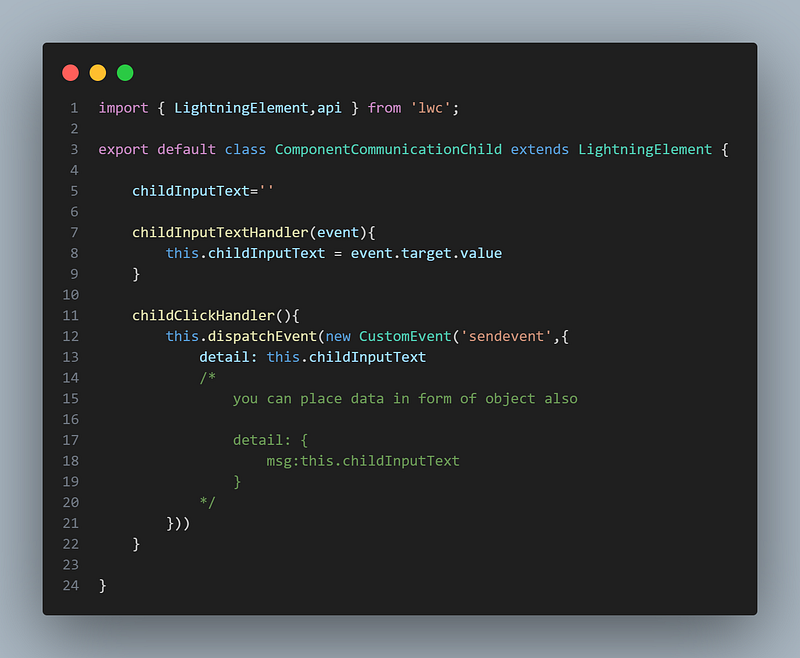
Result
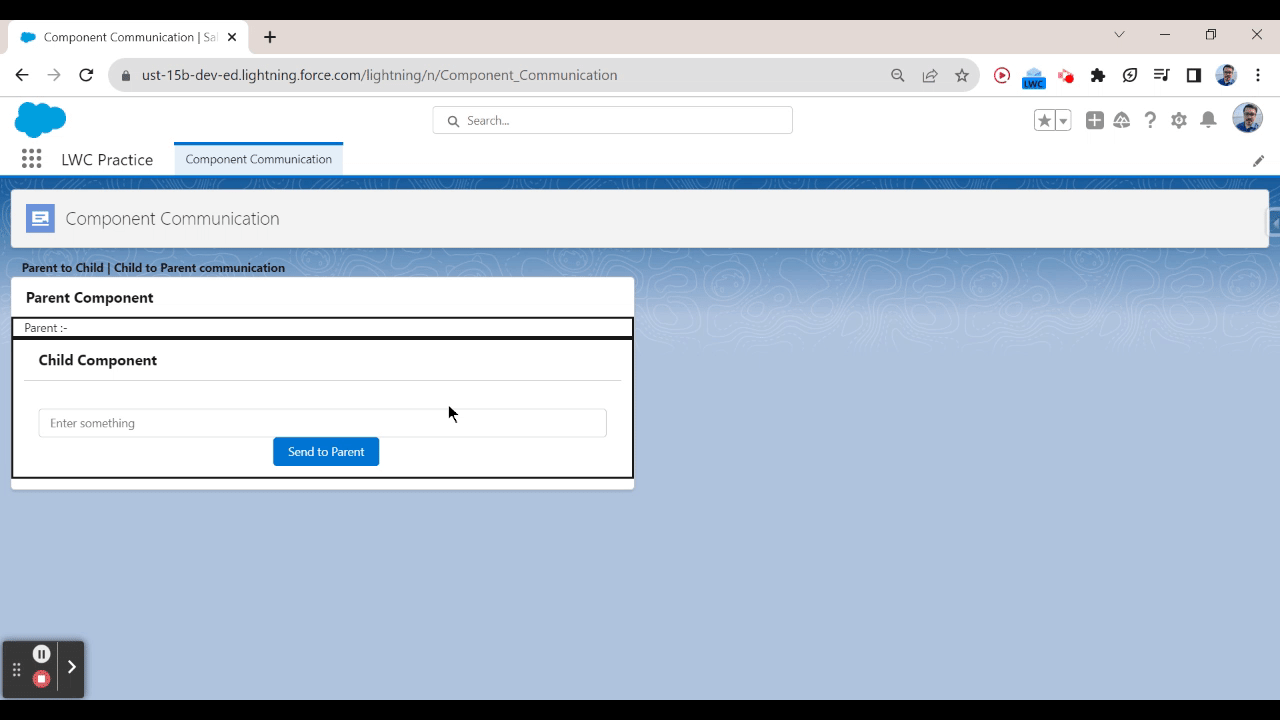
Independent Component communication using Pub-Sub Model
To achieve communication between two independent component, we use two techniques, 1) Pub-Sub model 2) Lightning Message Service (LMS)
In this topic we will learn how to make Independent Component Communication using Pub-Sub Model.
In a publish-subscribe pattern, one component publishes an event. Other components subscribe to receive and handle the event. Every component that subscribes to the event receives the event. The pubsub
module restricts events to a single page i.e you can perform communication between LWC to LWC only, if you wanna perform communicate across DOM, use LMS instead pub-sub model.
First, we need to make a component with any name depends on your choice, I have named as pubsub.
On that component, paste below code in your js file.
/* eslint-disable no-console */
const store = {};
/**
* subscribers a callback for an event
* @param {string} eventName - Name of the event to listen for.
* @param {function} callback - Function to invoke when said event is fired.
*/
const subscribe = (eventName, callback) => {
if (!store[eventName]) {
store[eventName] = new Set();
}
store[eventName].add(callback);
};
/**
* unsubscribe a callback for an event
* @param {string} eventName - Name of the event to unsubscribe from.
* @param {function} callback - Function to unsubscribe.
*/
const unsubscribe = (eventName, callback) => {
if (store[eventName]) {
store[eventName].delete(callback);
}
};
/**
* Publish an event to listeners.
* @param {string} eventName - Name of the event to publish.
* @param {*} payload - Payload of the event to publish.
*/
const publish = (eventName, payload) => {
if (store[eventName]) {
store[eventName].forEach(callback => {
try {
callback(payload);
} catch (error) {
console.error(error);
}
});
}
};
export default {
subscribe,
unsubscribe,
publish
};
In the above code, there are three Const variable which hold two paremeter, the first one is eventName and second is callback method
PubSubComp_A
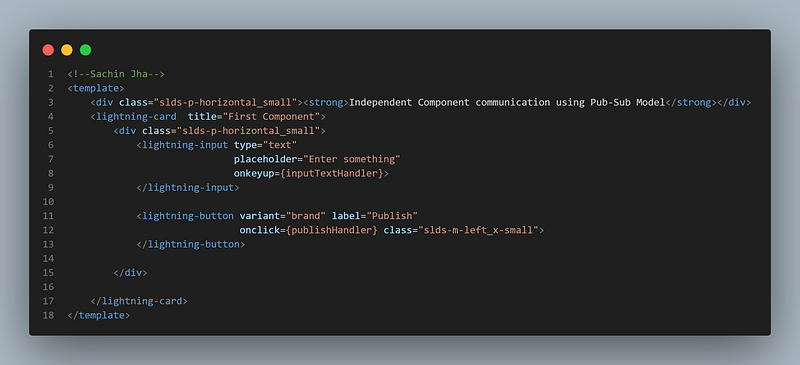
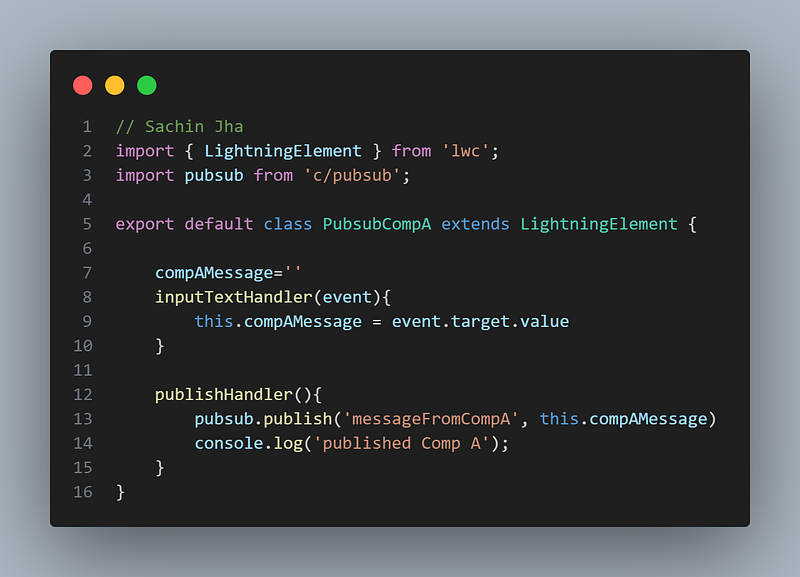
PubSubComp_B
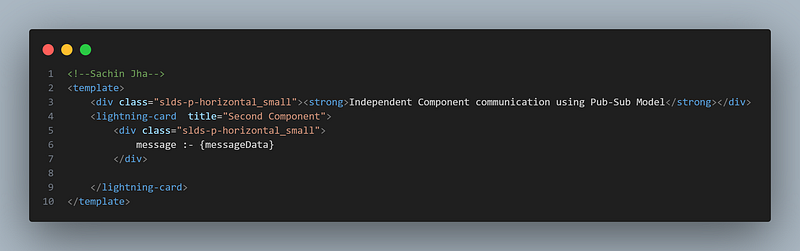
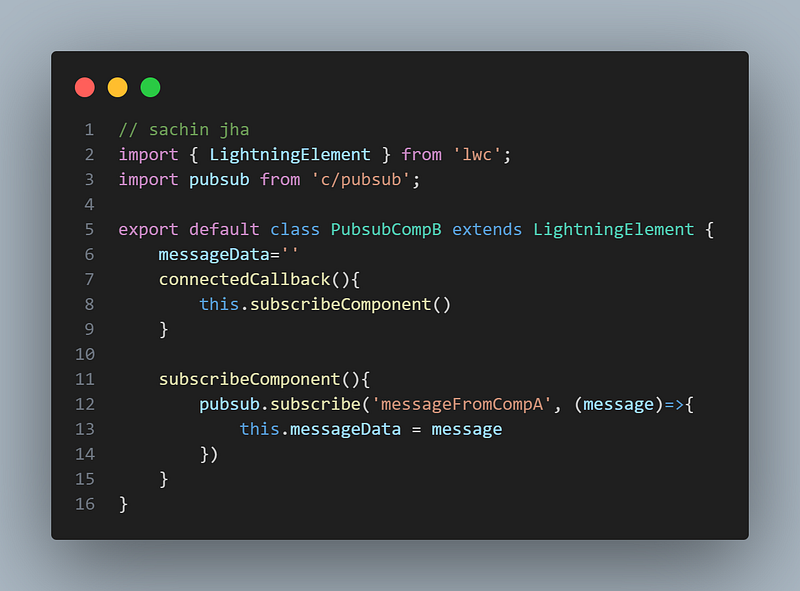
Result
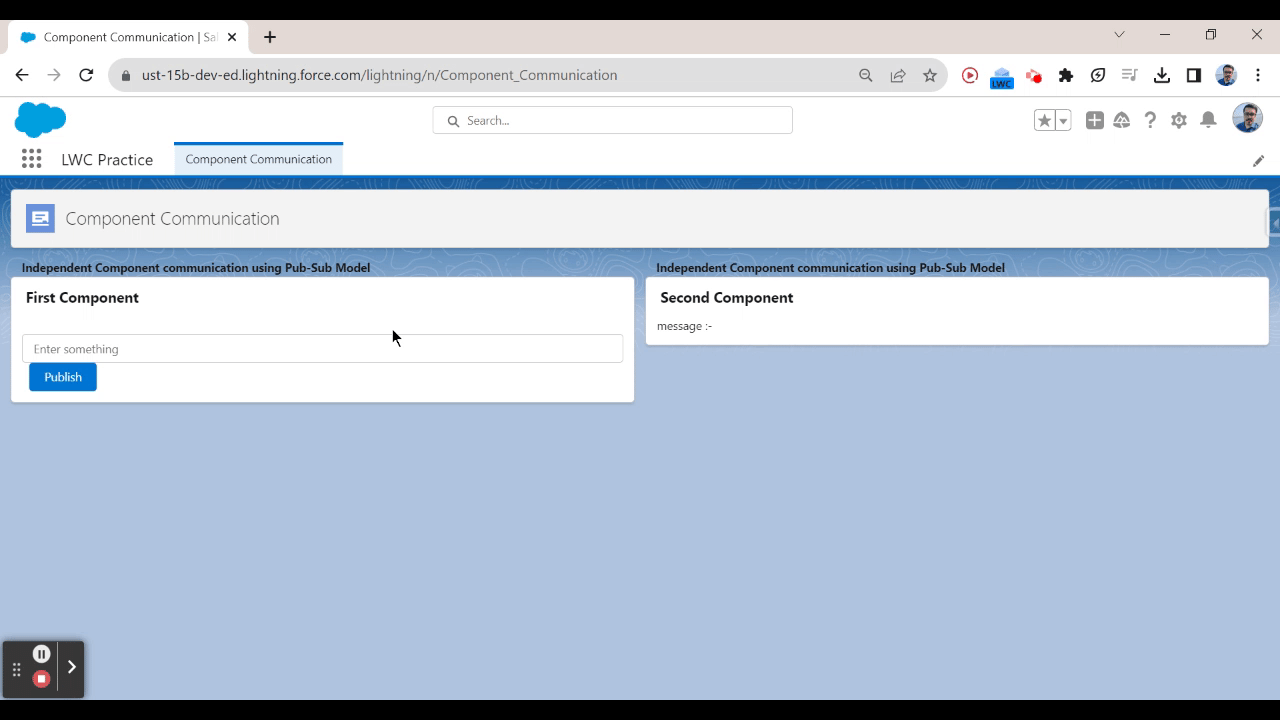
Follow me on linkedIn and Twitter for such more related post
Comments
Post a Comment