In this topic we will cover different way of Navigation used in LWC
Use the navigation service, lightning/navigation
, to navigate to many different page types, like records, list views, and objects.
In the component’s JavaScript class, import the NavigationMixin
function from the lightning/navigation
module.
import { NavigationMixin } from "lightning/navigation";
After importing you have to Apply the “NavigationMixin” function to your component’s base class
export default class MyCustomElement extends NavigationMixin(LightningElement) {}
Instead of a URL, the navigation service uses a PageReference
. A PageReference
is a JavaScript object that describes the page type, its attributes, and the state of the page.
PageReference Property
- type :- Its a String type, To navigate in Lightning Experience, Experience Builder sites, or the Salesforce mobile app, define a
PageReference
object. ThePageReference
type generates a unique URL format and defines attributes that apply to all pages of that type. - attributes :- Its a Object type, Map of name-value pairs that determine the target page. The page type defines the set of possible attribute names and their respective value types.
- state :- Its a Object type, Set of key-value pairs with string keys and string values. These parameters conditionally customize content within a given page. Some page reference types support a standard set of
state
properties.
The navigation service adds two APIs to your component’s class. Since these APIs are methods on the class, invoke them from this
.
▶[NavigationMixin.Navigate](pageReference, [replace])
A component callsthis[NavigationMixin.Navigate]
to navigate to another page in the application.
▶[NavigationMixin.GenerateUrl](pageReference)
A component callsthis[NavigationMixin.GenerateUrl]
to get apromise
that resolves to the resulting URL. The component can use the URL in thehref
attribute of an anchor. It can also use the URL to open a new window using thewindow.open({url})
browser API.
In this article, I have mentioned few Page References that are given below.
standard__namedPage
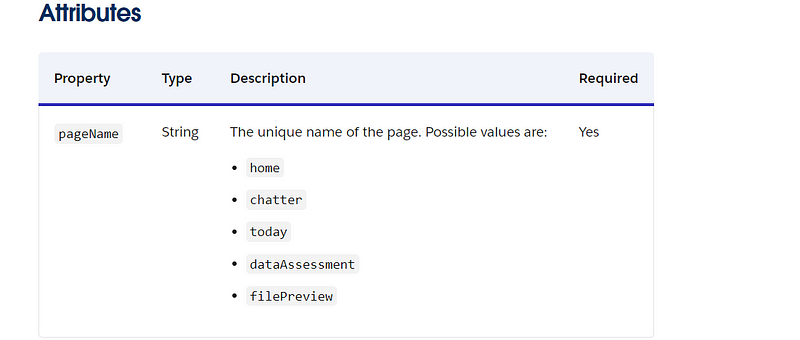
standard__objectPage
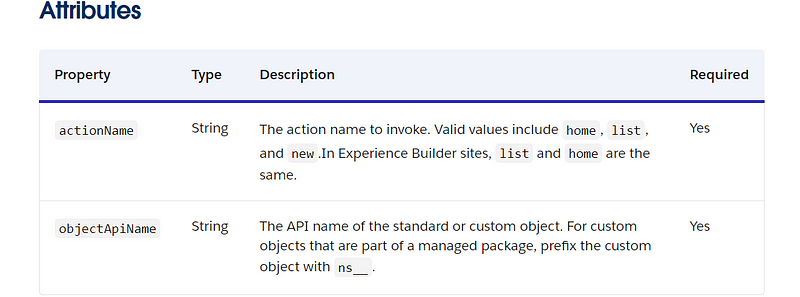
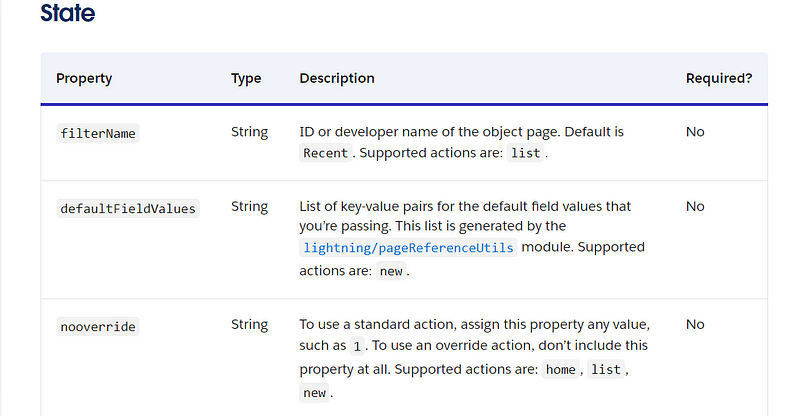
standard__navItemPage
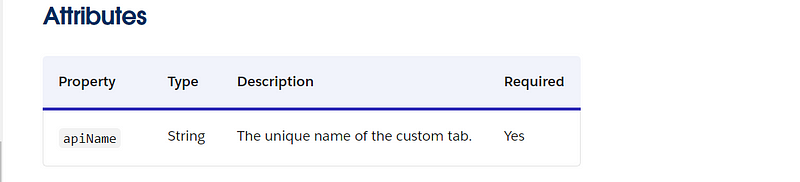
standard__recordPage
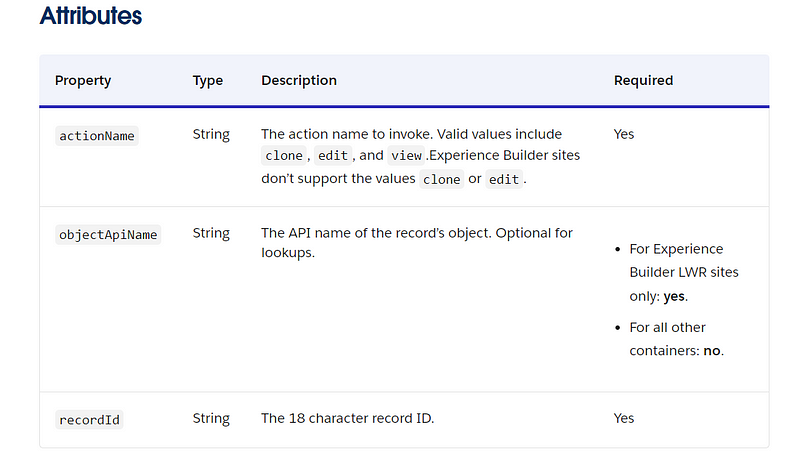
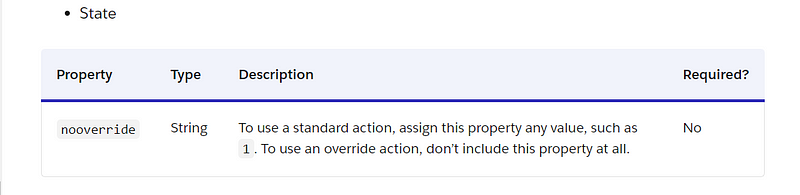
standard__recordRelationshipPage
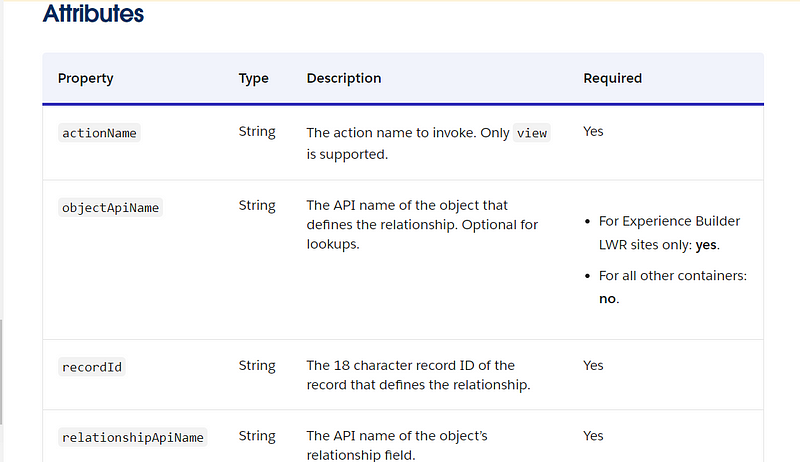
standard__webPage
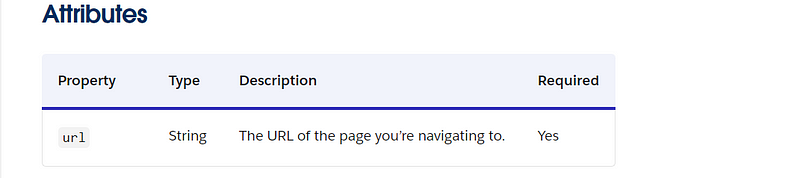
For more information about PageReference you can click here
Demonstration
Navigate to Home Page
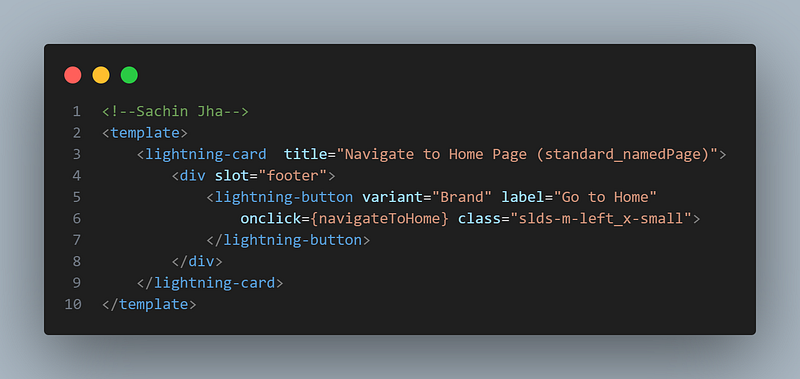
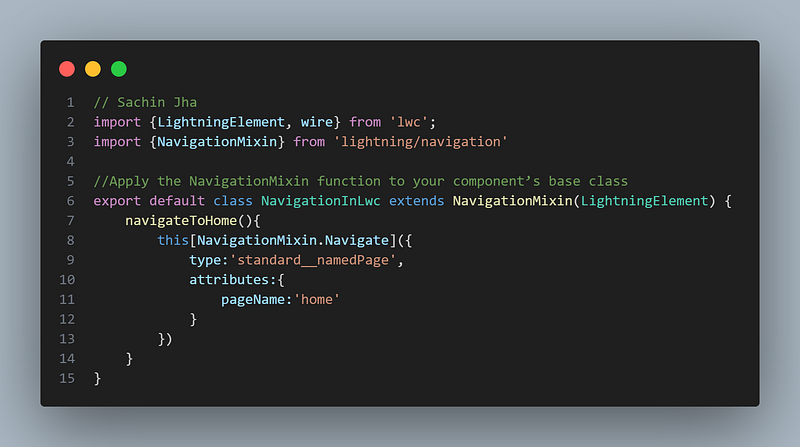
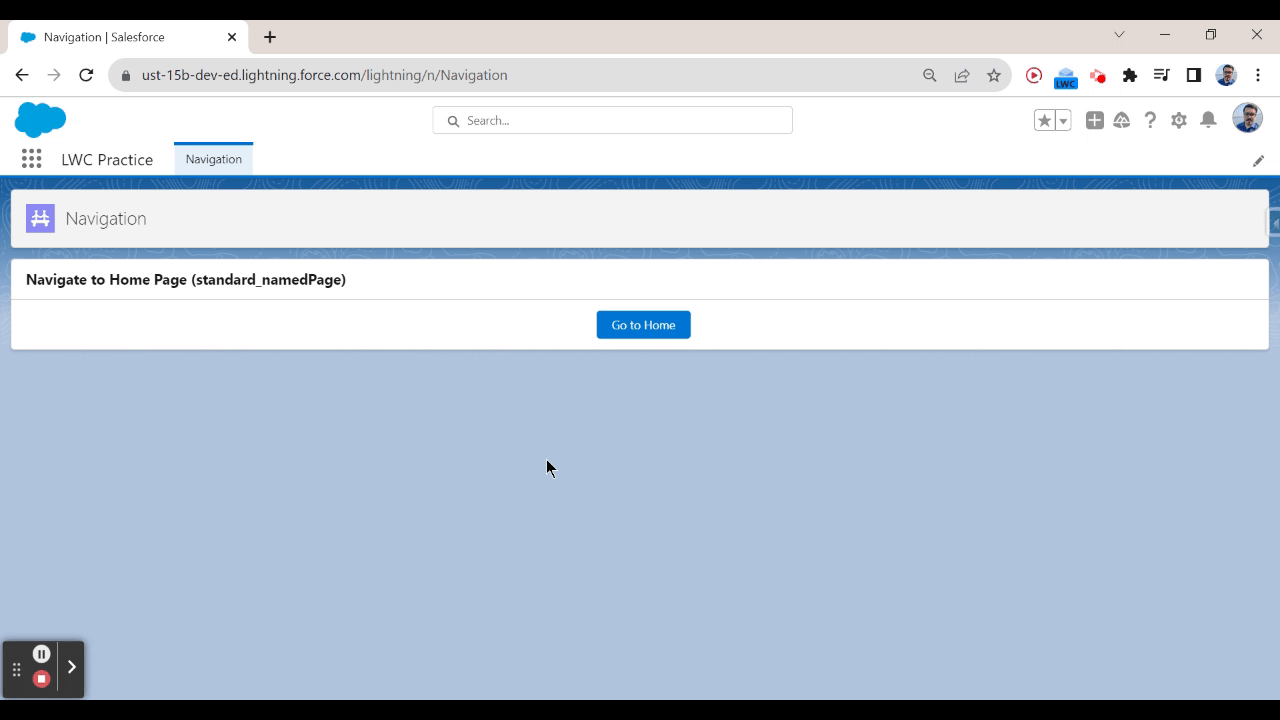
Navigate to Object Page
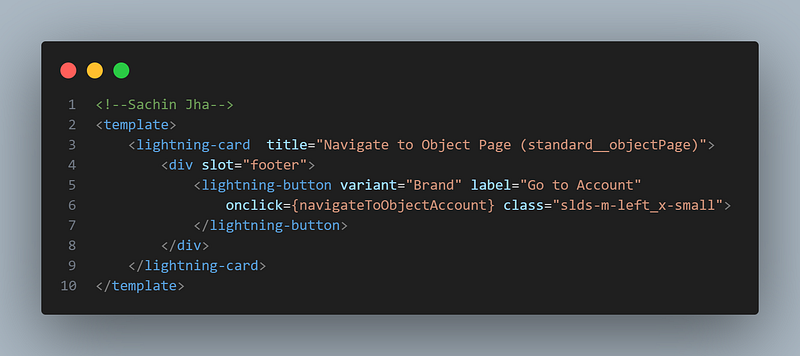
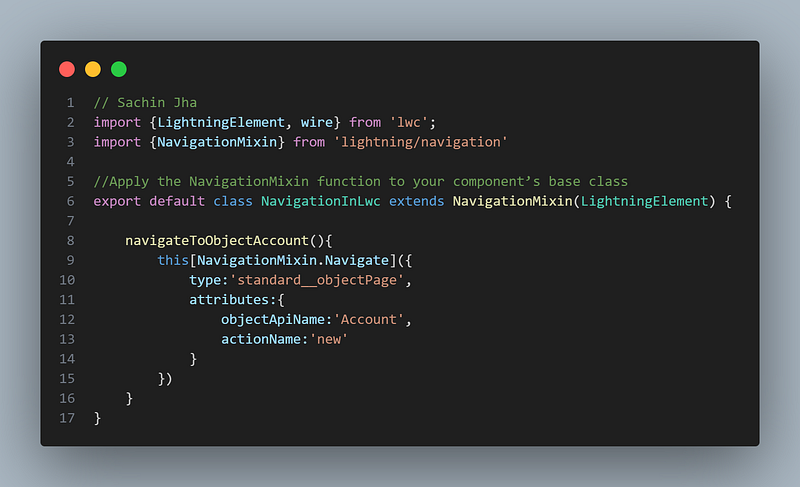
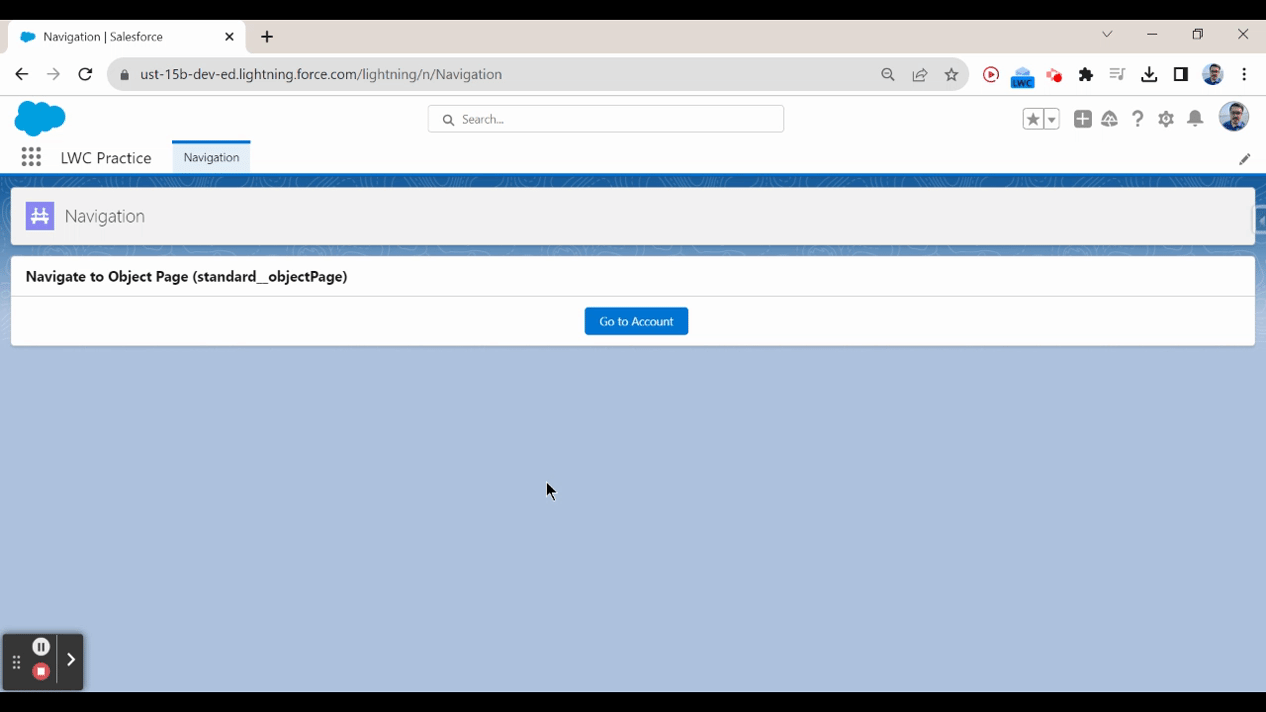
Navigate to Object page with default value
To provide a default value, we need to import encodeDefaultFieldValues from ‘lightning/pageReferenceUtils’.
import {encodeDefaultFieldValues} from 'lightning/pageReferenceUtils'
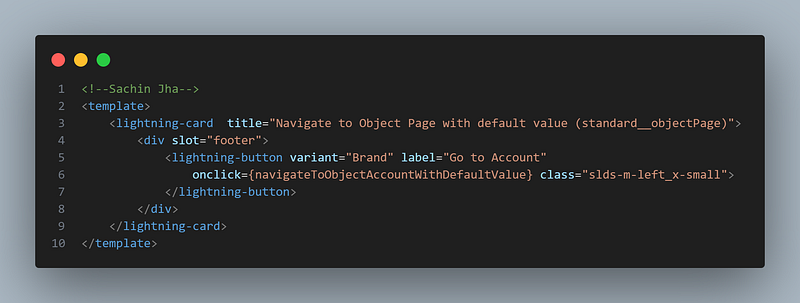
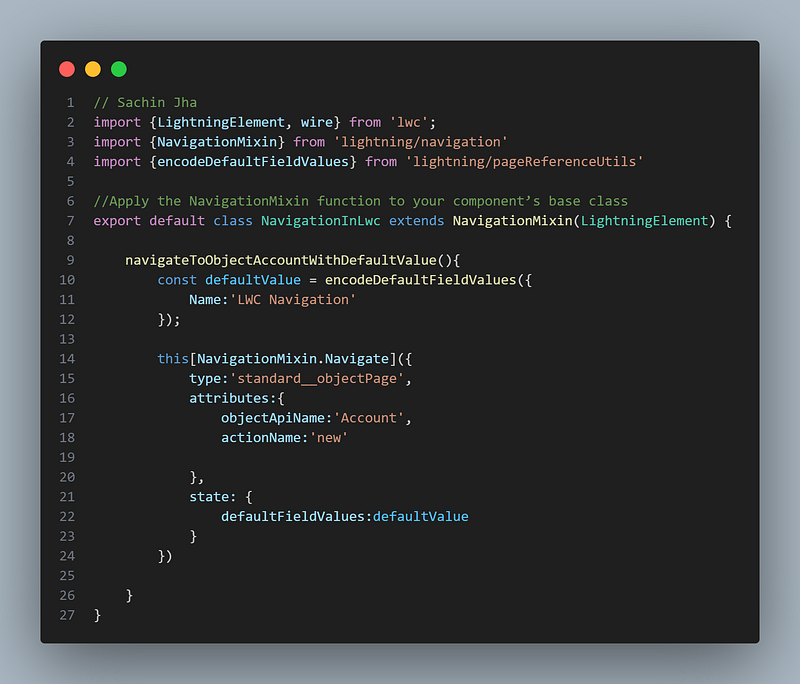
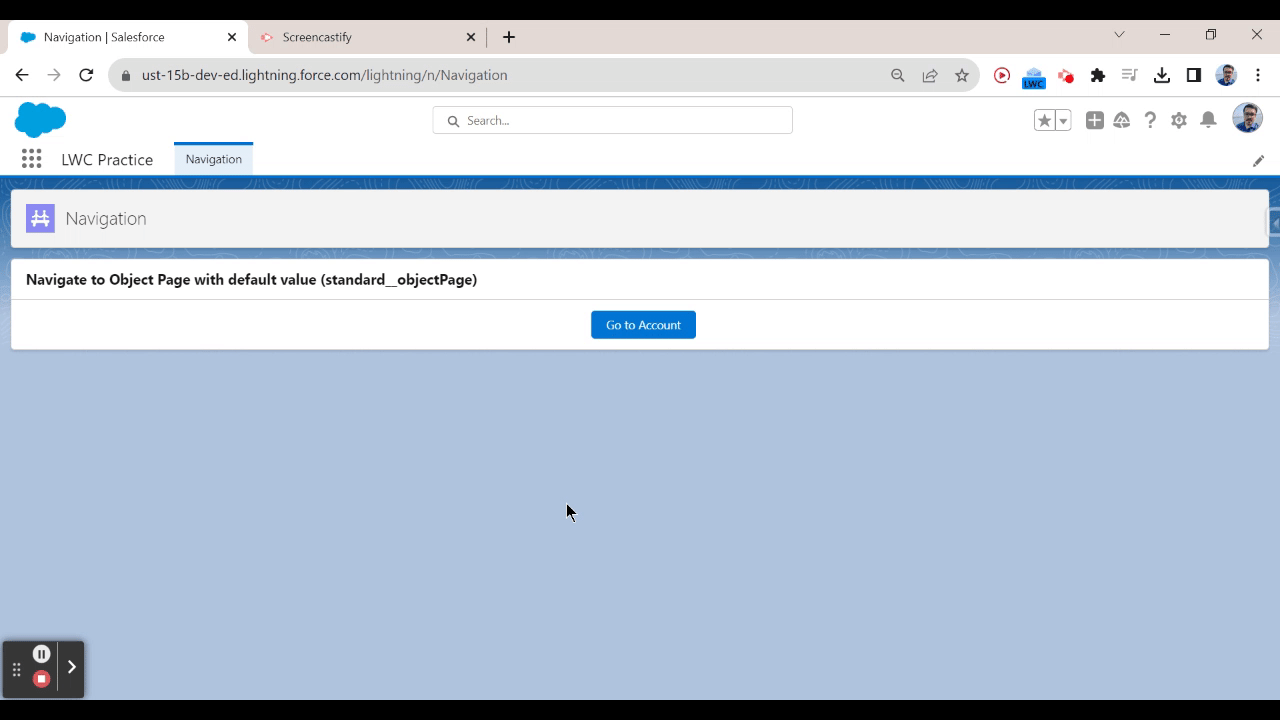
Navigate to Tab
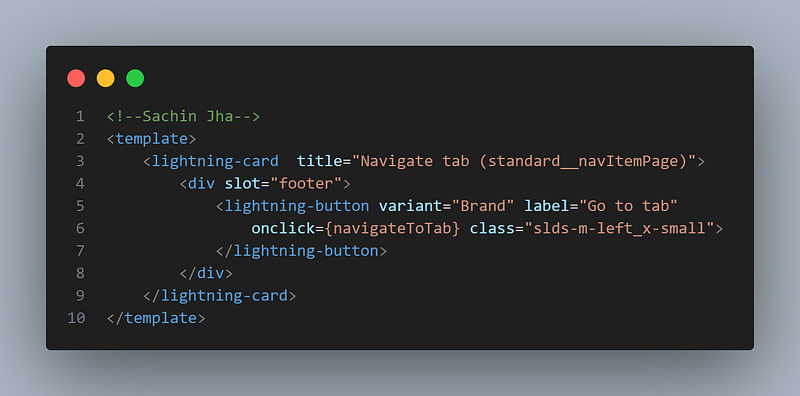
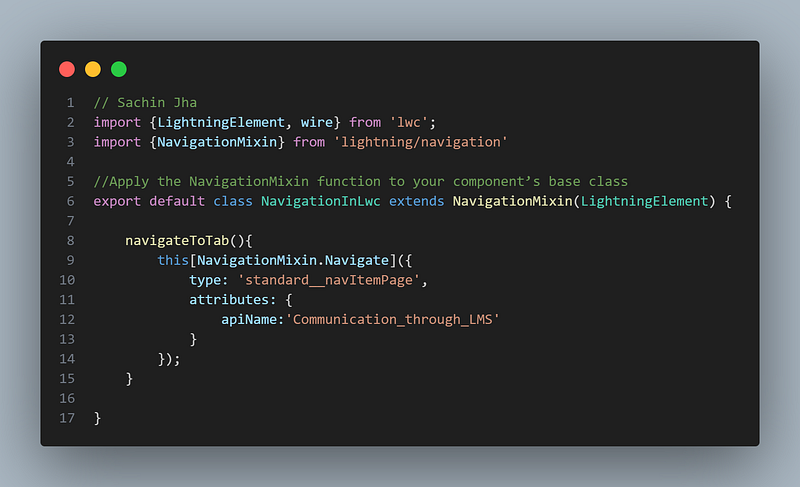
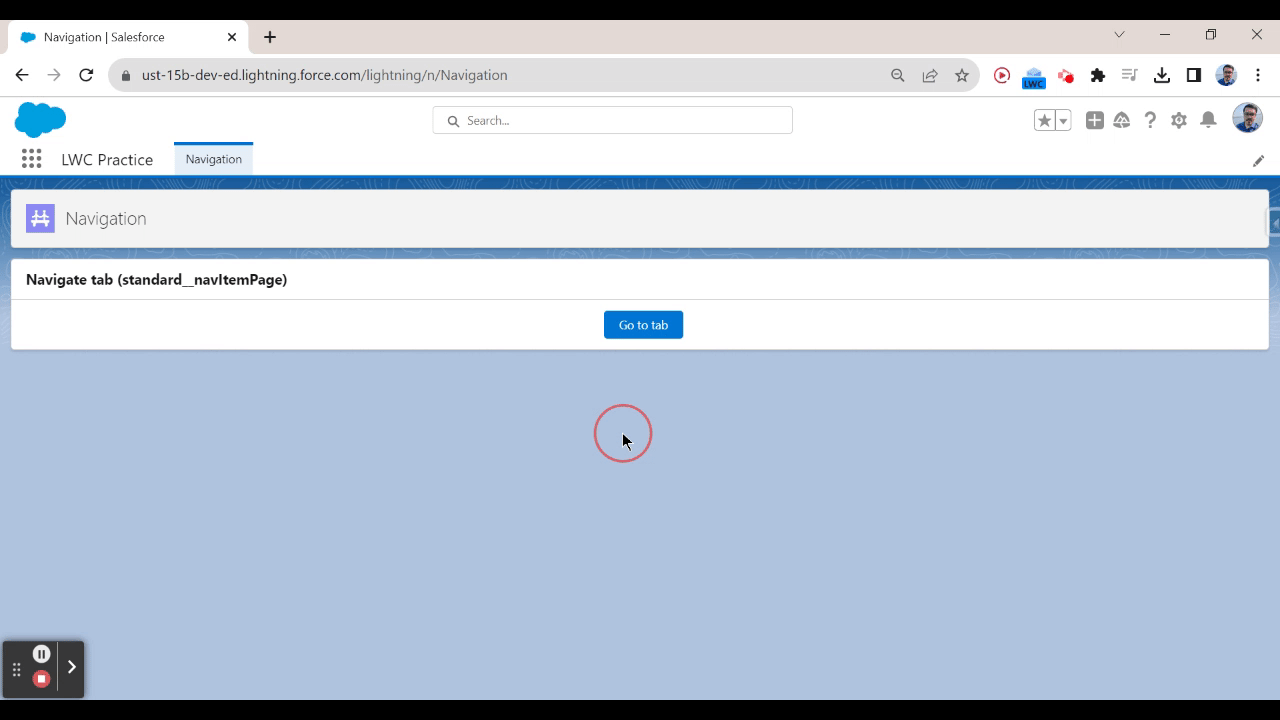
Navigate to Record Page
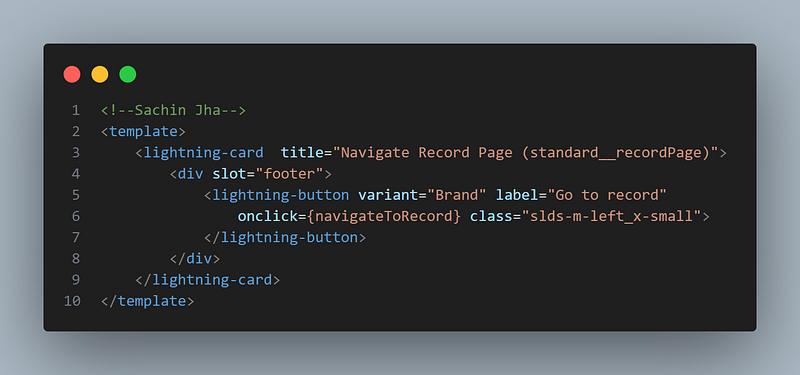
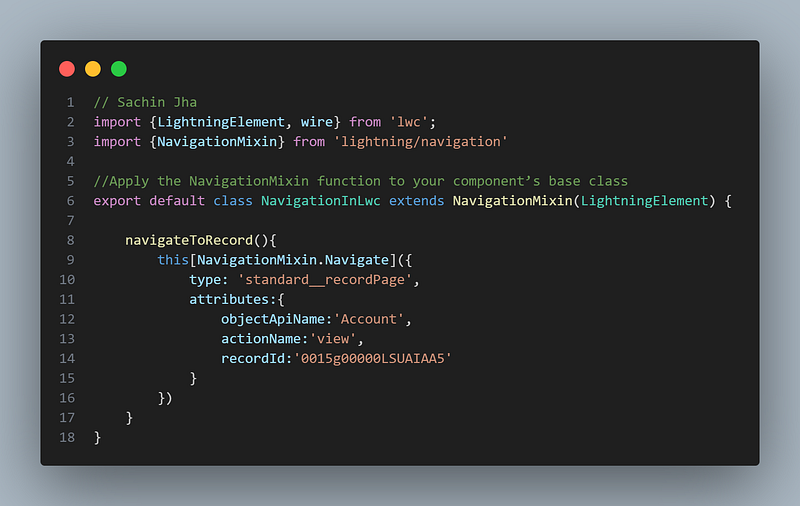
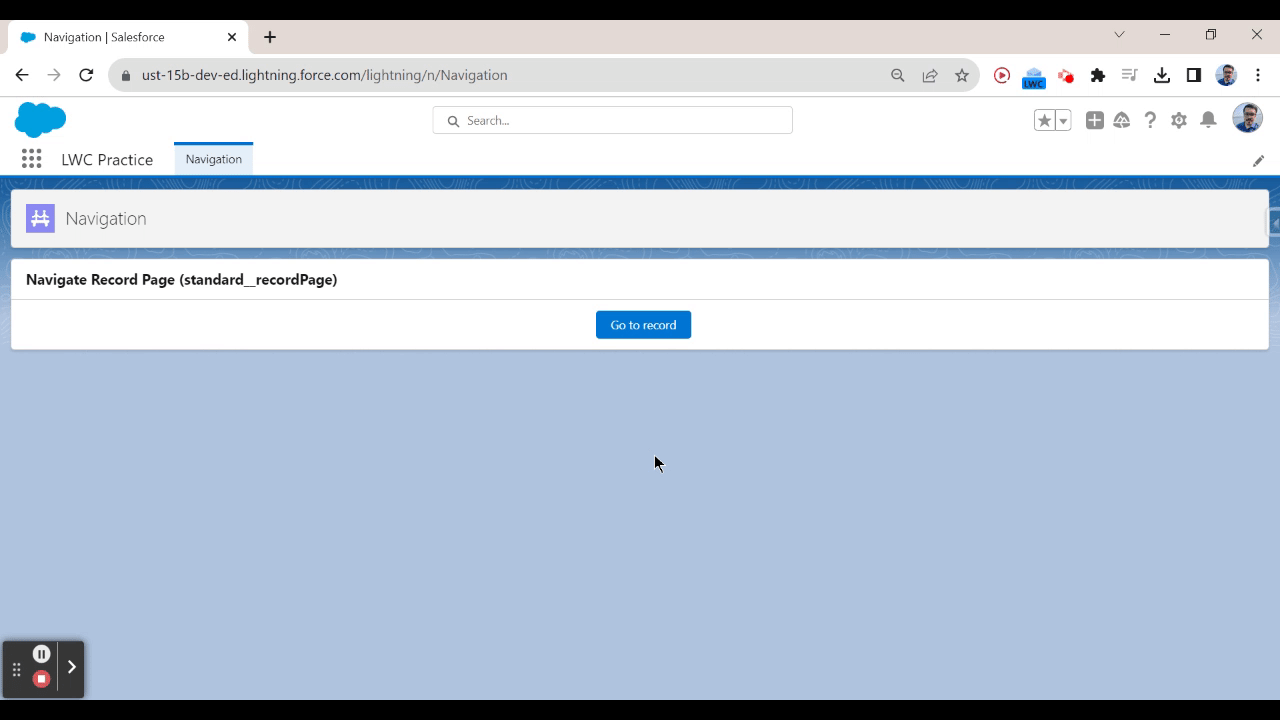
Navigate to Record Relationship Page
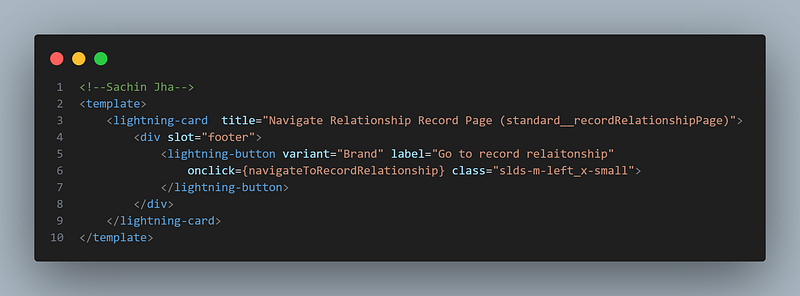
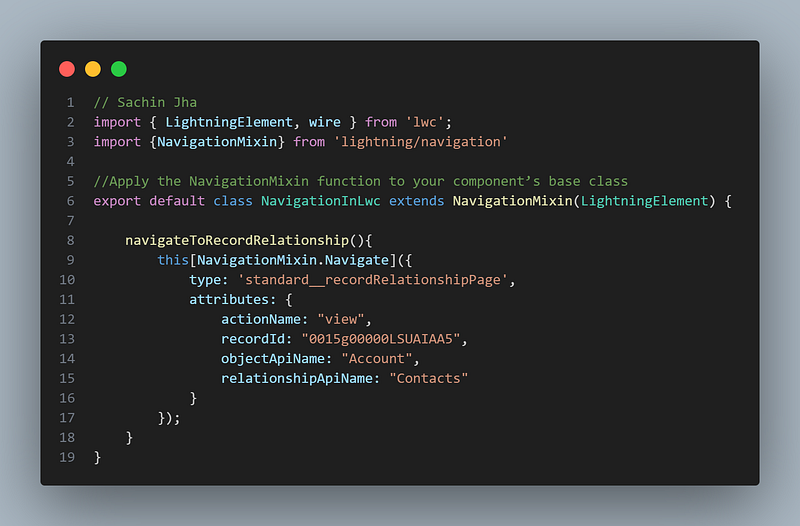
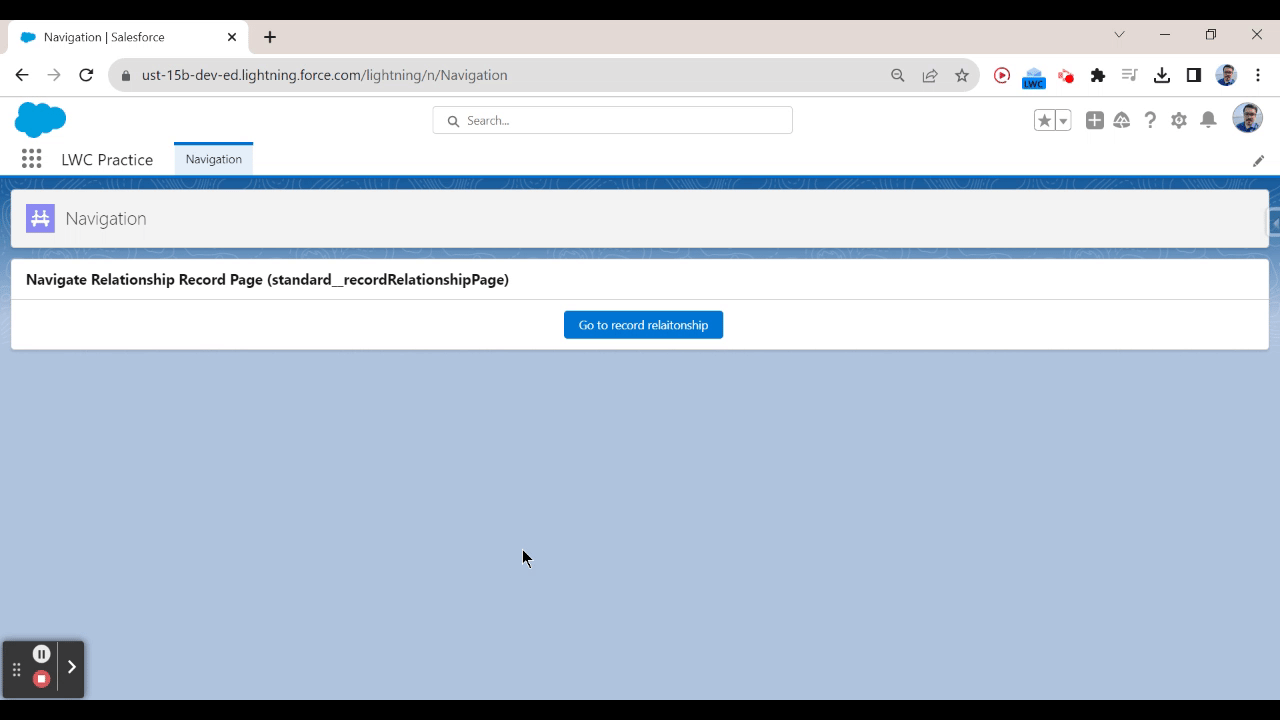
Navigate to External Web Page
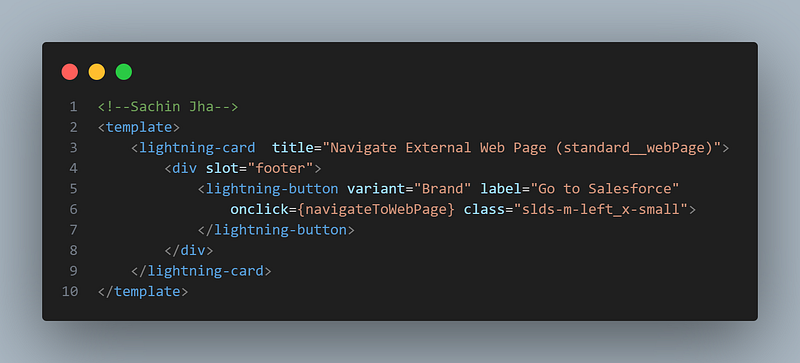
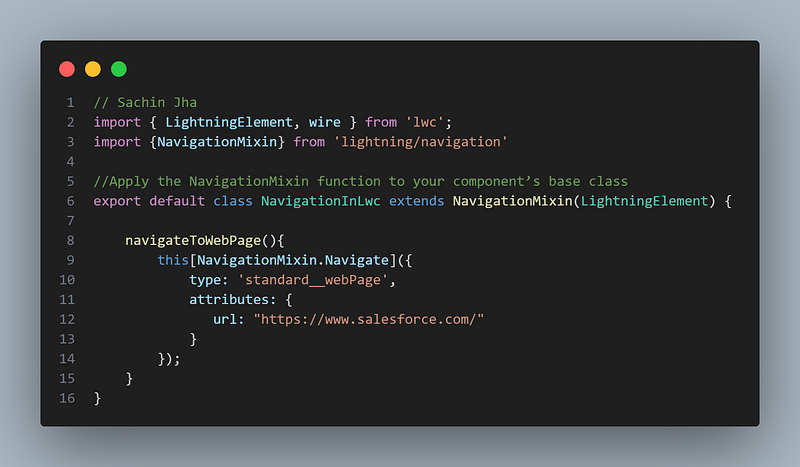
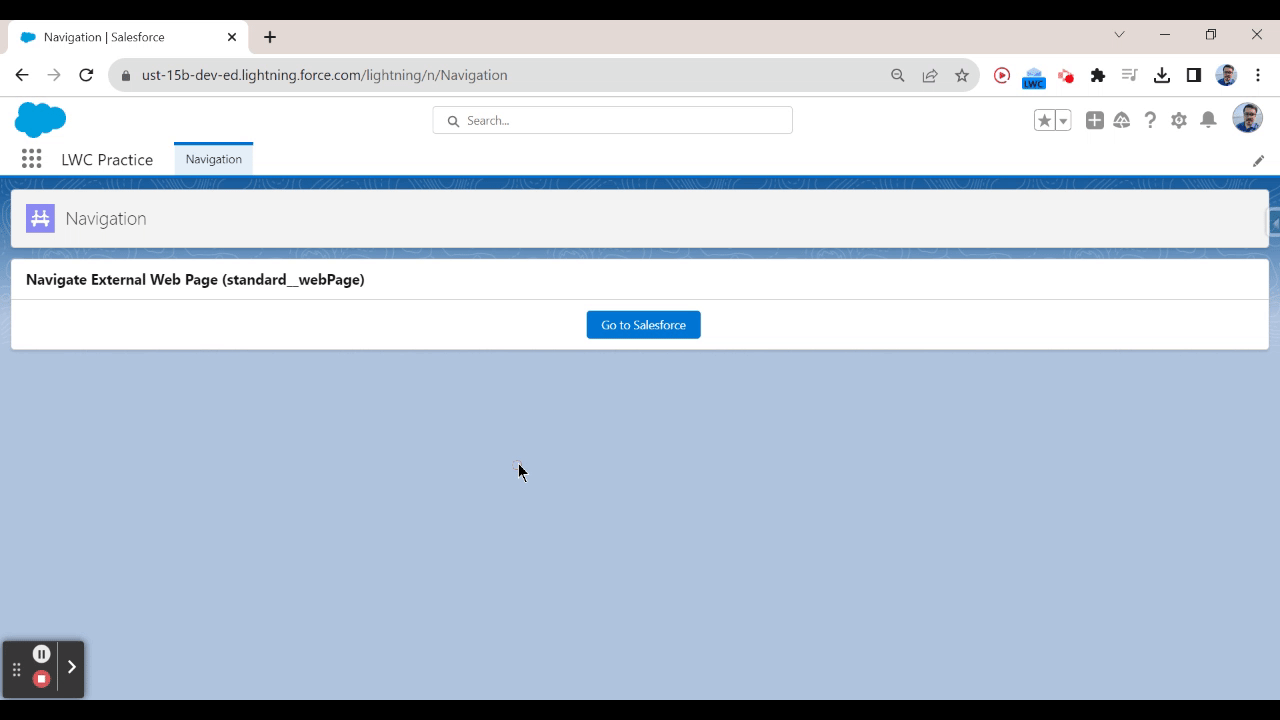
Navigate to LWC Component
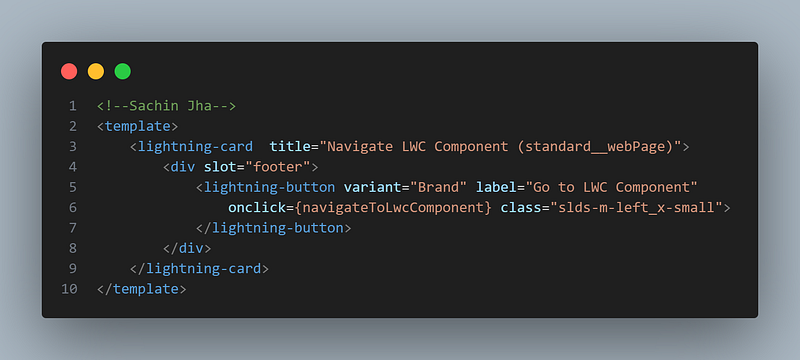
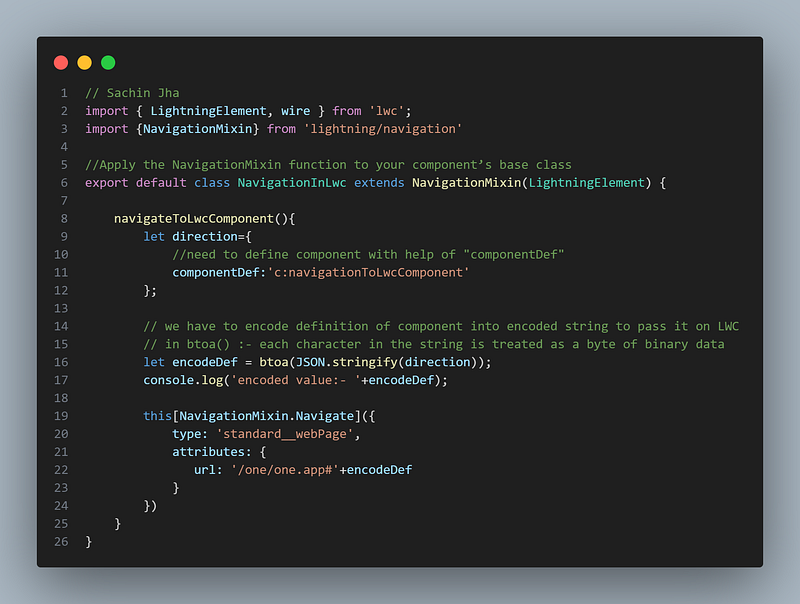
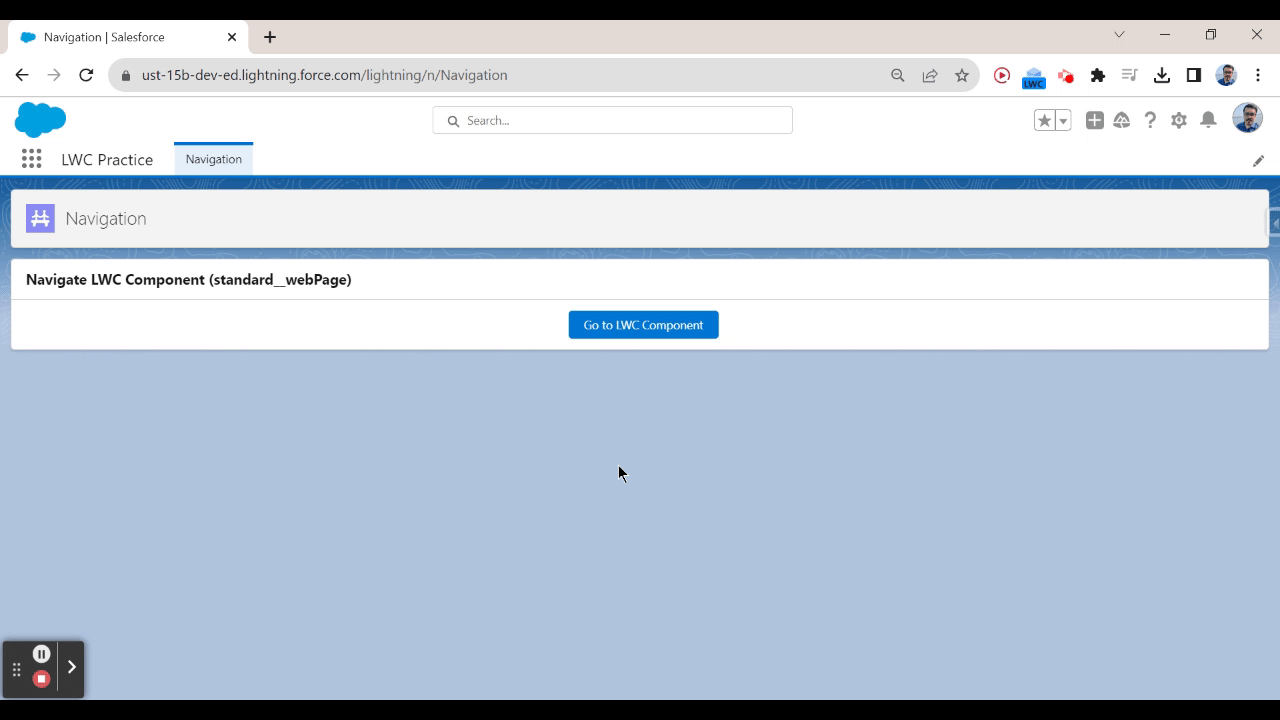
Get Current Page Reference
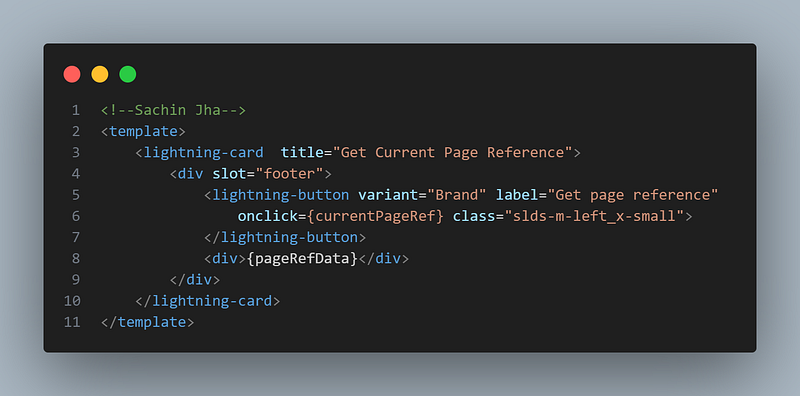
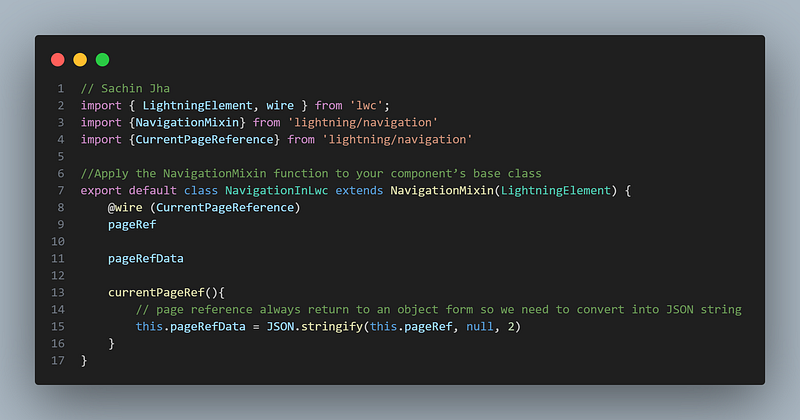
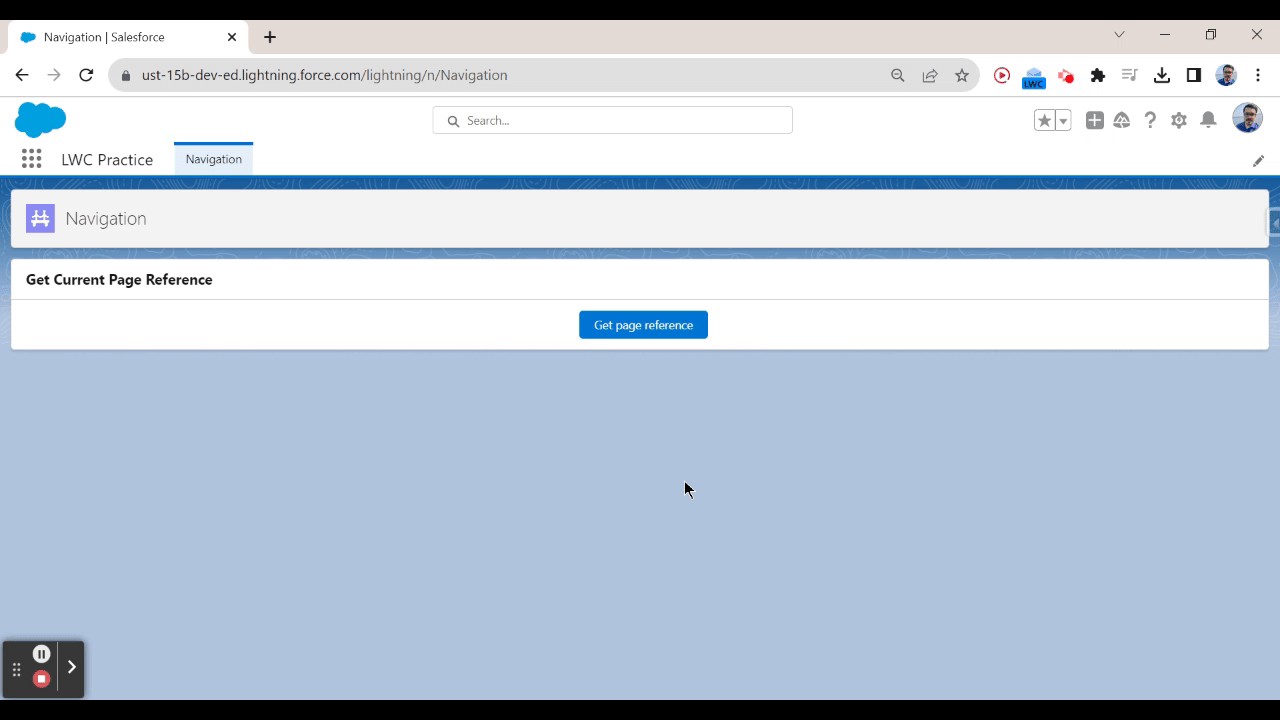
Follow me on linkedIn and Twitter for such more related post
Comments
Post a Comment